写出python使用OpenCV和深度学习模型YOLOv3进行图片中车辆检测的代码
时间: 2024-09-25 13:04:23 浏览: 9
要使用OpenCV(Open Source Computer Vision Library)结合YOLOv3(You Only Look Once version 3)在Python中进行图像中的车辆检测,你需要安装必要的库并理解YOLOv3的工作原理。首先,确保已经安装了以下库:
1. opencv-python:用于处理图像和视频
2. tensorflow或pytorch:用于深度学习模型
3. yolov3-tf2(如果使用TensorFlow)或yolov5(如果使用PyTorch):预训练的YOLOv3模型
这里是一个简单的示例,使用`yolov3-tf2`库,因为它是专为TensorFlow设计的。注意这只是一个基本框架,实际运行时可能需要根据你的具体需求调整参数。
```python
# 导入所需的库
import cv2
import os
from yolov3_tf2 import YOLOv3, load_yolo_weights
# 加载预训练的YOLOv3模型
model = YOLOv3(classes=80) # 假设车辆类别ID在列表的第80个位置
load_yolo_weights(model, 'path/to/yolov3.weights') # 替换为你的YOLOv3权重文件路径
# 定义主循环
def detect_vehicle(image_path):
# 读取图像
image = cv2.imread(image_path)
# 检测物体
boxes, scores, classes, _ = model.predict(image)
# 提取车辆检测结果
vehicle_boxes = [box for i, box in enumerate(boxes) if int(classes[i]) == 79] # 又假设车辆类别ID是79,替换为实际值
vehicle_scores = [scores[i] for i in range(len(scores)) if int(classes[i]) == 79]
# 对检测到的车辆框做非极大抑制(NMS)来去除重叠区域
indices = cv2.dnn.NMSBoxes(boxes, scores, 0.5, 0.4) # 阈值可以根据需要调整
# 绘制车辆检测框
for i in indices:
x, y, w, h = boxes[i]
label = f"Vehicle (score={vehicle_scores[i]:.2f})"
cv2.rectangle(image, (x, y), (x + w, y + h), color=(0, 255, 0), thickness=2)
cv2.putText(image, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示带有检测结果的图像
cv2.imshow("Vehicle Detection", image)
cv2.waitKey(0) # 按任意键关闭窗口
# 使用函数
image_path = "path/to/your/image.jpg"
detect_vehicle(image_path)
#
相关推荐
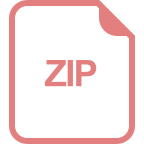
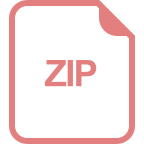
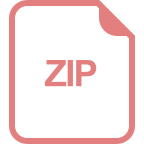
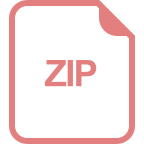
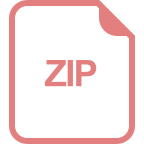
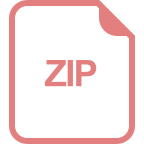
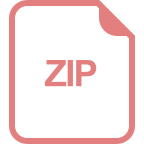
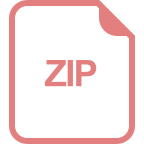
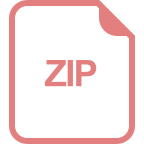
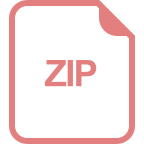
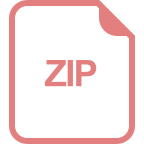
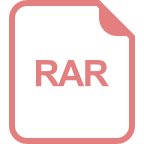
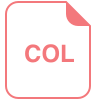




