mlp手写体数字识别mnist
时间: 2023-10-25 22:11:26 浏览: 115
MLP手写体数字识别MNIST是一种基于多层感知机(MLP)的图像分类算法,用于识别手写数字图像。MNIST数据集是一个常用的手写数字图像数据集,包含了60,000个训练样本和10,000个测试样本,每个样本都是28x28像素的灰度图像。MLP模型通过对这些图像进行训练,学习到了数字图像的特征,并能够对新的手写数字图像进行分类。除了MLP,还有其他的图像分类算法,如卷积神经网络(CNN)等。
相关问题
手写MLP的MINIST手写字体识别
抱歉,作为AI语言模型,我无法手写代码或进行图像识别任务。但是,我可以为您提供一个基本的MLP代码框架,您可以使用它来构建一个MINIST手写字体识别MLP模型:
```
import numpy as np
import matplotlib.pyplot as plt
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
# Define hyperparameters
learning_rate = 0.1
num_epochs = 100
batch_size = 100
# Define the number of neurons in each layer
input_layer = 784
hidden_layer1 = 256
hidden_layer2 = 256
output_layer = 10
# Define the input and output placeholders
X = tf.placeholder(tf.float32, [None, input_layer])
Y = tf.placeholder(tf.float32, [None, output_layer])
# Define the weights and biases for each layer
weights = {
'w1': tf.Variable(tf.random_normal([input_layer, hidden_layer1])),
'w2': tf.Variable(tf.random_normal([hidden_layer1, hidden_layer2])),
'out': tf.Variable(tf.random_normal([hidden_layer2, output_layer]))
}
biases = {
'b1': tf.Variable(tf.random_normal([hidden_layer1])),
'b2': tf.Variable(tf.random_normal([hidden_layer2])),
'out': tf.Variable(tf.random_normal([output_layer]))
}
# Define the forward propagation
hidden_layer1_output = tf.nn.relu(tf.add(tf.matmul(X, weights['w1']), biases['b1']))
hidden_layer2_output = tf.nn.relu(tf.add(tf.matmul(hidden_layer1_output, weights['w2']), biases['b2']))
output = tf.add(tf.matmul(hidden_layer2_output, weights['out']), biases['out'])
# Define the loss function and optimizer
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=output, labels=Y))
optimizer = tf.train.GradientDescentOptimizer(learning_rate=learning_rate).minimize(loss)
# Define the accuracy metric
correct_pred = tf.equal(tf.argmax(output, 1), tf.argmax(Y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
# Initialize the variables
init = tf.global_variables_initializer()
# Start the session
with tf.Session() as sess:
sess.run(init)
num_batches = int(mnist.train.num_examples/batch_size)
# Train the model
for epoch in range(num_epochs):
avg_loss = 0
for batch in range(num_batches):
batch_x, batch_y = mnist.train.next_batch(batch_size)
_, l = sess.run([optimizer, loss], feed_dict={X: batch_x, Y: batch_y})
avg_loss += l/num_batches
if epoch % 10 == 0:
print("Epoch:", epoch+1, " Loss:", avg_loss)
# Test the model
test_accuracy = sess.run(accuracy, feed_dict={X: mnist.test.images, Y: mnist.test.labels})
print("Test Accuracy:", test_accuracy)
```
这个代码框架包含了一个基本的MLP模型,使用MNIST数据集进行训练和测试。您可以根据需要进行调整和修改,以获得更好的性能和准确性。
阅读全文
相关推荐
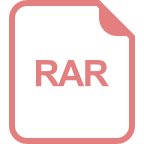
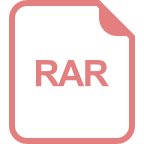
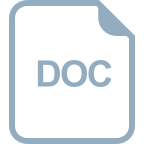
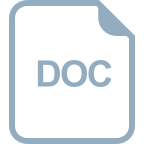
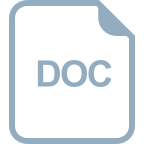
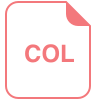
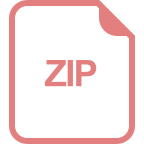
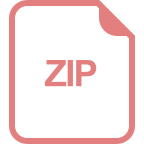
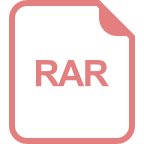
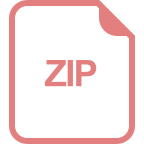
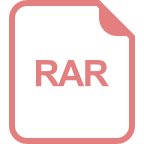
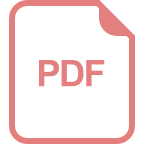
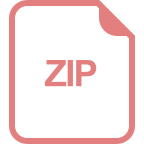
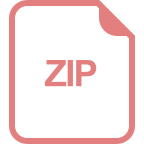
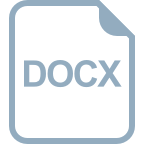
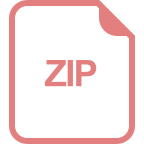