元胞自动机 交通流时空分布图 代码
时间: 2024-12-27 19:24:14 浏览: 4
### 关于元胞自动机模拟交通流时空分布图的代码实现
元胞自动机模型被广泛应用于交通流的研究中,通过定义简单的局部规则来描述复杂的宏观现象。对于交通流而言,可以利用一维或二维网格表示道路网络,在每个时间步更新车辆的状态。
#### 基础概念
在构建此类仿真程序之前,需理解几个核心要素:
- **格子空间**:通常采用线性链表结构代表单向车道上的位置序列;
- **演化法则**:基于特定条件调整速度并移动到下一时刻的新位点;
这些原则有助于创建基本框架[^1]。
#### Python 实现示例
下面给出一段简化版的一维纳什沃尔夫(Nagel-Schreckenberg)模型Python代码用于展示如何建立这样的系统:
```python
import numpy as np
import matplotlib.pyplot as plt
class TrafficFlowSimulation:
def __init__(self, length=200, density=0.3, max_speed=5, p_slowdown=0.3):
self.length = length # 道路长度
self.density = density # 车辆密度
self.max_speed = max_speed # 最大允许车速
self.p_slowdown = p_slowdown # 减速概率
num_cars = int(self.length * self.density)
positions = sorted(np.random.choice(range(length), size=num_cars, replace=False))
speeds = list(map(lambda _: min(int(np.random.rand()*(max_speed+1)), max_speed), range(num_cars)))
self.cars = [(pos, speed) for pos, speed in zip(positions, speeds)]
def update_positions(self):
new_positions = []
updated_cars = []
for i, (position, velocity) in enumerate(sorted(self.cars)):
next_pos = position + velocity
if next_pos >= self.length:
continue
front_distance = None
for j in range(i+1, len(self.cars)+i):
idx = j % len(self.cars)
other_position, _ = self.cars[idx]
dist = ((other_position - next_pos) + self.length) % self.length
if dist != 0 and (front_distance is None or dist < front_distance):
front_distance = dist
if front_distance is not None:
desired_velocity = min(front_distance-1, self.max_speed)
actual_velocity = min(max(velocity-1, 0),
desired_velocity,
velocity + int(np.random.rand()>self.p_slowdown))
final_pos = position + actual_velocity
while any((final_pos-pos)%self.length==0 for pos,_ in updated_cars):
final_pos += 1
new_positions.append(final_pos%self.length)
updated_cars.append((final_pos%self.length,actual_velocity))
self.cars = updated_cars
def visualize(self, steps=100):
fig, ax = plt.subplots()
images = []
for step in range(steps):
img_data = ['.'*self.length]*len(self.cars)
for car_idx,(car_pos,speed) in enumerate(self.cars):
row = car_idx//int(len(img_data)**0.5)
col = car_idx-(row*int(len(img_data)**0.5))
img_data[row]=img_data[row][:col]+'X'+img_data[row][col+1:]
image=ax.imshow([list(s.replace(' ',''))for s in img_data], cmap='binary')
images.append([image])
self.update_positions()
ani = animation.ArtistAnimation(fig, images, interval=50, blit=True, repeat_delay=1000)
plt.show()
if __name__ == "__main__":
sim = TrafficFlowSimulation(density=.2,max_speed=5,p_slowdown=.4)
sim.visualize(steps=200)
```
此脚本实现了基础版本的一维NS模型,并提供了可视化功能以便观察随时间变化而形成的模式。注意这只是一个非常初级的例子,实际应用可能需要更复杂的功能和优化措施。
阅读全文
相关推荐
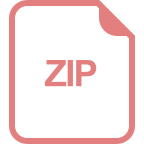
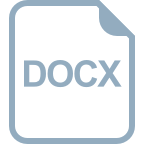
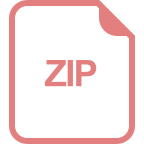
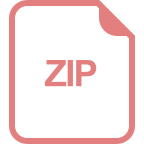
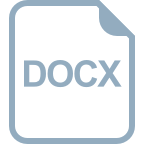
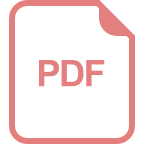
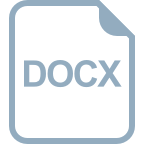
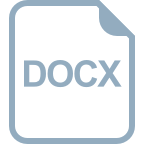
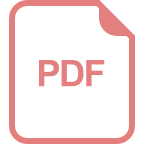
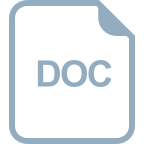

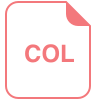
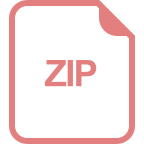
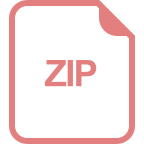
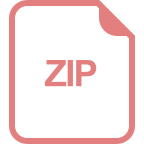
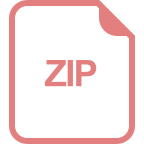
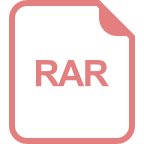