java基础案例4-3
时间: 2024-09-28 10:04:27 浏览: 9
在Java基础教程中,案例4-3通常涉及创建一个简单的"Shape"(形状)抽象类和它的两个子类,比如"Circle"(圆形)和"Squre"(正方形)。这个例子展示了面向对象编程中的基本概念,如封装、继承和多态。
```java
// Shape抽象类
abstract class Shape {
// 定义抽象方法
abstract void draw();
}
// Circle子类
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
void draw() {
System.out.println("Drawing a circle with radius " + radius);
}
}
// Square子类
class Square extends Shape {
private double side;
public Square(double side) {
this.side = side;
}
@Override
void draw() {
System.out.println("Drawing a square with side length " + side);
}
}
public class Main {
public static void main(String[] args) {
// 创建并绘制实例
Shape circle = new Circle(5);
circle.draw(); // 输出: Drawing a circle with radius 5
Shape square = new Square(10);
square.draw(); // 输出: Drawing a square with side length 10
}
}
```
在这个案例中,`draw()`方法被重写并在各自的子类中实现了不同的功能,体现了多态性。在`main`函数中,虽然引用的是`Shape`类型的变量,但是通过实例化不同的子类,我们可以绘制出不同的形状。
相关推荐
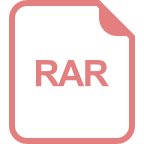
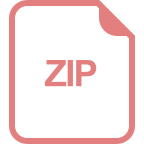














