c语言编写程序,用户只输入一个8位2进制数,要求对每位之间进行异或运算,最终输出0或1
时间: 2024-09-10 22:08:14 浏览: 51
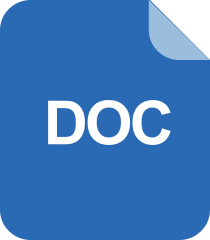
c语言位运算c语言位运算
在C语言中,你可以通过以下步骤编写一个简单的程序,让用户输入一个8位二进制数,并对其各位进行异或(XOR)操作:
```c
#include <stdio.h>
#include <bitset>
// Function to perform XOR operation on binary digits
void xor_digits(unsigned char input)
{
std::bitset<8> bitset(input); // Convert input to a bitset for easy manipulation
std::bitset<8> result = bitset; // Create a copy of the bitset
// Perform XOR operation between each pair of bits
for (size_t i = 1; i < 7; ++i) {
result[i] ^= bitset[i]; // XOR current bit with next bit
}
// If final result is all zeros or all ones, output 0, otherwise output 1
if (result.to_ulong() == 0 || result.to_ulong() == 0xFF) {
printf("Output: 0\n");
} else {
printf("Output: 1\n");
}
}
int main()
{
unsigned char binary_num;
printf("Enter an 8-bit binary number: ");
scanf("%1x", &binary_num); // Read user input as hexadecimal, which represents an 8-bit number
// Validate input
if (binary_num >= 0 && binary_num <= 0xFF) {
xor_digits(binary_num);
} else {
printf("Invalid input! Please enter an 8-bit binary number.\n");
}
return 0;
}
```
这个程序首先将用户输入的8位二进制数转换为`std::bitset`方便处理。然后,它逐个对每一位进行异或操作。最后,检查结果是否全为0或全为1,如果是,则输出0,否则输出1。
阅读全文
相关推荐
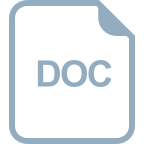
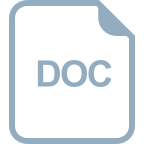


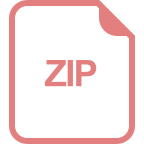
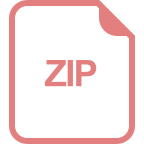
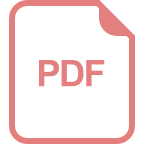
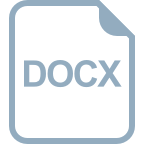
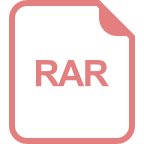
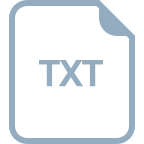
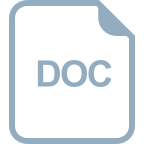
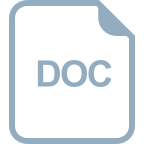
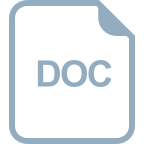
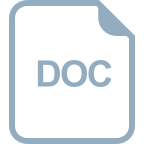
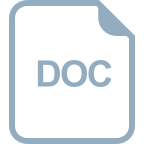
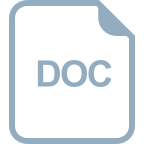
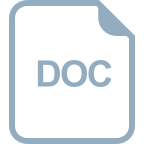
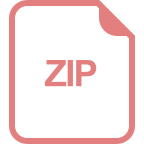