遗传算法求香蕉函数的极大值 python实现
时间: 2024-01-15 20:05:09 浏览: 125
以下是使用遗传算法求解香蕉函数极大值的Python实现:
```python
import random
# 定义香蕉函数
def rosenbrock(x1, x2):
return (1 - x1)**2 + 100 * (x2 - x1**2)**2
# 定义遗传算法
def genetic_algorithm(Size, G, Codel, umax, umin, pc, pm):
# 初始化种群
population = []
for _ in range(Size):
individual = [random.uniform(umin, umax) for _ in range(Codel)]
population.append(individual)
# 迭代进化
for _ in range(G):
# 计算适应度
fitness = [rosenbrock(individual[0], individual[1]) for individual in population]
# 选择
selected_population = []
for _ in range(Size):
# 轮盘赌选择
total_fitness = sum(fitness)
pick = random.uniform(0, total_fitness)
current_fitness = 0
for i in range(Size):
current_fitness += fitness[i]
if current_fitness > pick:
selected_population.append(population[i])
break
# 交叉
offspring_population = []
for i in range(0, Size, 2):
parent1 = selected_population[i]
parent2 = selected_population[i+1]
if random.random() < pc:
# 单点交叉
crossover_point = random.randint(1, Codel-1)
offspring1 = parent1[:crossover_point] + parent2[crossover_point:]
offspring2 = parent2[:crossover_point] + parent1[crossover_point:]
else:
offspring1 = parent1
offspring2 = parent2
offspring_population.append(offspring1)
offspring_population.append(offspring2)
# 变异
for i in range(Size):
individual = offspring_population[i]
for j in range(Codel):
if random.random() < pm:
individual[j] = random.uniform(umin, umax)
# 更新种群
population = offspring_population
# 找到最优解
best_fitness = float('inf')
best_individual = None
for individual in population:
fitness = rosenbrock(individual[0], individual[1])
if fitness < best_fitness:
best_fitness = fitness
best_individual = individual
return best_fitness, best_individual[0], best_individual[1]
# 调用遗传算法求解香蕉函数的极大值
Size = 100 # 种群大小
G = 100 # 迭代次数
Codel = 2 # 编码长度
umax = 2 # 变量上界
umin = -2 # 变量下界
pc = 0.8 # 交叉概率
pm = 0.1 # 变异概率
BestSfi, BestS, x1, x2 = genetic_algorithm(Size, G, Codel, umax, umin, pc, pm)
print("Best fitness: ", BestSfi)
print("Best solution: ", BestS)
print("x1: ", x1)
print("x2: ", x2)
```
阅读全文
相关推荐
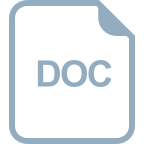

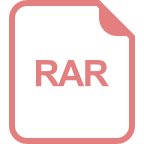
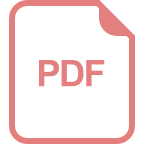
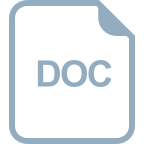
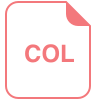
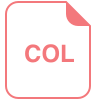
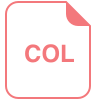
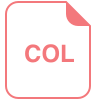
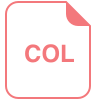
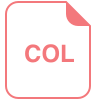
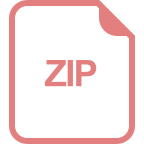
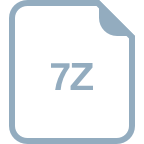