给定一个长度为n的成绩表(成绩表中包含学生的姓名、成绩), 以及一个整数k, 请设计一个算法求出成绩表从小到大排序后的第k个学生的姓名、成绩(成绩保留到小数点后1位),并列则全部输出。用c语言写出
时间: 2024-12-15 19:29:43 浏览: 7
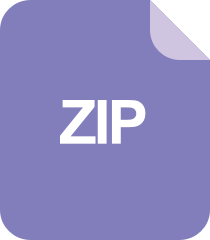
链表学生成绩管理系统
为了实现这个功能,你可以使用优先队列(堆)数据结构。C语言标准库中没有内置的优先队列,但可以自定义一个最小堆来达到目的。这里是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h> // 用于操作内存和创建堆
// 结构体定义成绩条目
typedef struct {
char name[50];
float score;
} Student;
// 比较函数,用于堆排序
int compare(const void *a, const void *b) {
const Student *sa = (const Student *)a;
const Student *sb = (const Student *)b;
return sa->score - sb->score; // 按分数降序排列
}
// 自定义最小堆
typedef struct {
int size;
Student *data;
} MinHeap;
void min_heapify(MinHeap *heap, int index) {
int left = 2 * index + 1;
int right = 2 * index + 2;
int smallest = index;
if (left < heap->size && compare(heap->data + left, heap->data + index) < 0)
smallest = left;
if (right < heap->size && compare(heap->data + right, heap->data + smallest) < 0)
smallest = right;
if (smallest != index) {
swap(heap->data + index, heap->data + smallest);
min_heapify(heap, smallest);
}
}
void insert(MinHeap *heap, const Student *student) {
heap->data = realloc(heap->data, sizeof(Student) * (heap->size + 1));
heap->data[heap->size] = *student;
heap->size++;
min_heapify(heap, heap->size - 1);
}
Student* find_kth_student(MinHeap *heap, int k) {
while (heap->size > k) {
heap->size--;
min_heapify(heap, 0);
}
if (heap->size == k) {
return heap->data;
} else {
return NULL; // 如果k超过堆大小,表示不存在这样的学生
}
}
// 主函数
int main() {
MinHeap heap;
heap.size = 0;
heap.data = malloc(sizeof(Student));
// 假设scores数组存储了学生的姓名和成绩
Student scores[] = {{"张三", 89.5}, {"李四", 95.0}, {"王五", 87.0}};
int n = sizeof(scores) / sizeof(scores[0]);
for (int i = 0; i < n; i++) {
insert(&heap, &scores[i]);
}
int k;
scanf("%d", &k);
Student *result = find_kth_student(&heap, k);
if (result) {
printf("第 %d 个学生的姓名: %s, 成绩: %.1f\n", k, result->name, result->score);
} else {
printf("找不到第%d个学生。\n", k);
}
free(heap.data);
return 0;
}
```
这个程序首先创建了一个最小堆,然后将成绩表插入堆中,并保持堆的特性。当查询第k个学生时,通过调整堆的大小找到对应的索引。注意,这个代码假定输入的k值不会导致堆溢出。
阅读全文
相关推荐
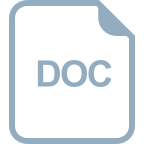
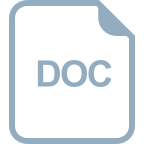





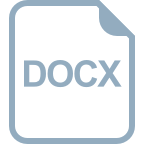
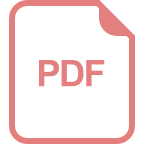
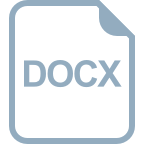
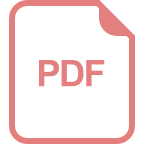
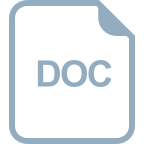





