c# 传递用户名密码访问共享文件服务器上的文件
时间: 2024-10-09 16:11:10 浏览: 74
在C#中,为了通过用户名和密码访问共享文件服务器上的文件,通常会涉及到网络文件系统(Network File System,NFS)或Windows网络共享功能。你可以使用`System.IO.Packaging`库来操作网络文件,或者直接通过`System.Net.WebClient`或`System.Net.Sockets`进行低级操作。这里是一个基本的步骤说明:
1. **使用WebClient**:
```csharp
using System.Net;
using System.IO;
string userName = "your_username";
string password = "your_password";
string serverPath = "\\server_address\\shared_folder";
var client = new WebClient();
client.Credentials = new NetworkCredential(userName, password);
try {
byte[] fileBytes = client.DownloadData(serverPath);
// 将文件字节数组保存到本地或其他位置
File.WriteAllBytes("local_file_path", fileBytes);
} catch (Exception ex) {
Console.WriteLine($"Error accessing file: {ex.Message}");
}
```
2. **使用DirectoryInfo和FileStream**:
```csharp
using System.IO;
using System.Security.Principal;
string username = "your_username";
string password = "your_password";
string filePath = "\\server_address\\shared_folder\\file.txt";
// 创建一个Principal对象用于身份验证
var identity = new GenericPrincipal(new GenericIdentity(username), null);
// 使用Credentials获取安全上下文
var credentials = new NetworkCredential(username, password);
var securityContext = new SafeHandleInheritable(credentials.GetCredential(NetworkAuthentication.DefaultHost, "NTLM").GetNativeObject(), true);
using (FileStream fs = File.OpenRead(filePath, FileAccess.Read, FileShare.Read))
using (Stream networkStream = new NetworkStream(fs, securityContext)) {
// 读取文件内容...
}
```
注意:这些示例假设你正在使用Windows环境,并且共享文件服务器支持NTLM或Kerberos认证。在实际项目中,还需要考虑异常处理和权限管理。
阅读全文
相关推荐






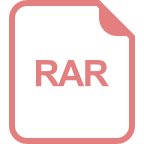

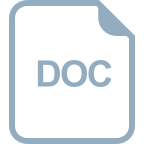
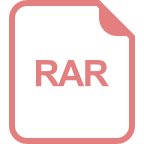

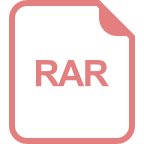

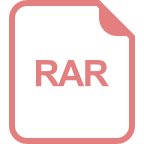
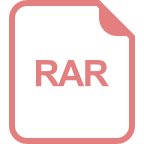


