java 使用BaseMapper 插入数据后,怎么返回自增id
时间: 2024-10-23 10:06:51 浏览: 76
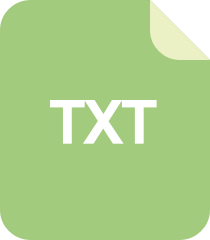
ID自增JAVA类方法

在Java中,使用MyBatis(基于Java的持久层框架)和BaseMapper接口进行数据插入时,如果你想要获取自增ID(通常用于主键),你需要做如下几步:
1. **映射文件配置**:在`mapper.xml`文件中,对于包含自增长ID的字段,指定其`useGeneratedKeys="true"`以及`keyProperty`属性,比如:
```xml
<insert id="insert" parameterType="com.example.YourEntity">
INSERT INTO your_table (field1, field2)
VALUES (#{field1}, #{field2})
<if test="your_entity.id == null">
.generatedKey property="id"
</if>
</insert>
```
2. **实体类配置**:在对应的实体类`YourEntity`中,添加`@GeneratedValue(strategy=GenerationType.AUTO)`注解,表示使用数据库的自增策略生成ID,同时声明`private Long id;`作为属性。
3. **BaseMapper接口**:在BaseMapper接口中定义一个方法来返回插入后的ID,MyBatis会在后台为你处理这个请求:
```java
@Insert("insert into your_table (field1, field2) values(#{field1}, #{field2})")
@Options(useGeneratedKeys = true, keyProperty = "id")
public int insertWithId(YourEntity entity);
```
4. **业务逻辑**:在实际的业务服务中,调用上述方法并将结果保存到实体对象中:
```java
YourEntity newEntity = new YourEntity();
// 设置其他字段...
int rowsInserted = mapper.insertWithId(newEntity);
if (rowsInserted > 0) {
newEntity.setId(newEntity.getId()); // 可能为null,直到事务提交后才有效
}
```
阅读全文
相关推荐
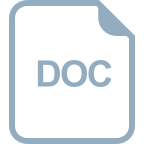
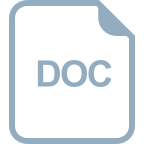


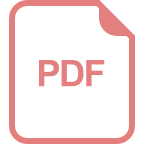
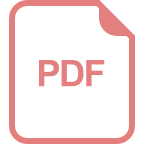
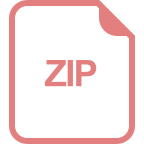
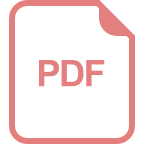
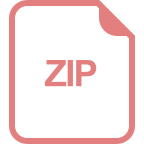
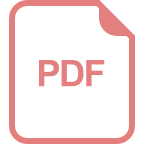
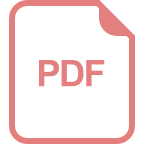
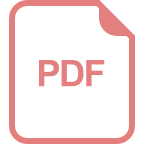
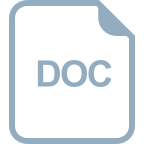
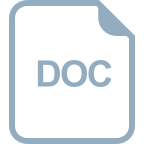



