pytorch实现使用CNN及空洞卷积预测中医开方的中药品种和每个中药的剂量
时间: 2024-04-22 13:26:17 浏览: 187
下面是一个使用 PyTorch 实现 CNN 及空洞卷积(Dilated Convolution)进行中医开方预测的简单示例:
```python
import torch
import torch.nn as nn
class MedicinePrescriptionCNN(nn.Module):
def __init__(self, num_classes):
super(MedicinePrescriptionCNN, self).__init__()
self.conv1 = nn.Conv1d(in_channels=1, out_channels=64, kernel_size=3, padding=1)
self.conv2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=3, padding=1, dilation=2)
self.fc1 = nn.Linear(128, 64)
self.fc2 = nn.Linear(64, num_classes)
def forward(self, x):
x = self.conv1(x)
x = nn.functional.relu(x)
x = self.conv2(x)
x = nn.functional.relu(x)
x = torch.mean(x, dim=2)
x = self.fc1(x)
x = nn.functional.relu(x)
x = self.fc2(x)
return x
# 示例用法
num_classes = 10 # 分类的中药品种数
batch_size = 16
seq_length = 100 # 每个中药的序列长度
input_channels = 1 # 输入通道数(因为我们使用的是灰度图像,所以通道数为 1)
model = MedicinePrescriptionCNN(num_classes)
# 创建一个随机输入张量作为示例
input_tensor = torch.randn(batch_size, input_channels, seq_length)
# 前向传播
output = model(input_tensor)
# 输出预测结果的形状
print(output.shape) # 输出: torch.Size([16, 10])
```
在这个示例中,我们定义了一个名为 `MedicinePrescriptionCNN` 的模型,它包含了两个卷积层(使用了不同的空洞率)和两个全连接层。模型的输入是一个大小为 `(batch_size, input_channels, seq_length)` 的张量,其中 `batch_size` 是批量大小,`input_channels` 是输入通道数,`seq_length` 是每个中药的序列长度。模型的输出是一个预测分类结果的张量,形状为 `(batch_size, num_classes)`。
希望这个示例能够帮助你理解如何使用 CNN 及空洞卷积进行中医开方预测。如果你有任何其他问题,请随时提问!
阅读全文
相关推荐
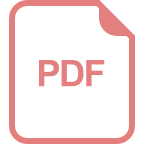
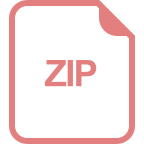
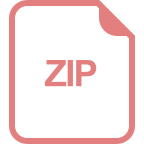
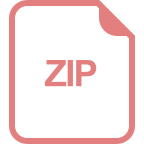
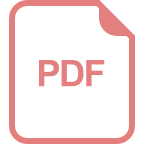
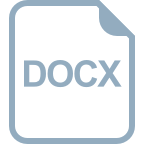
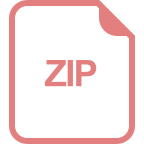
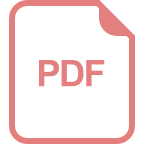
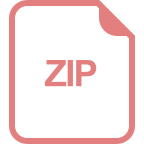
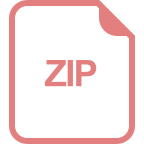
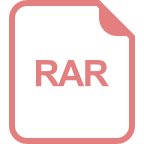
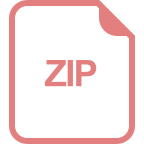
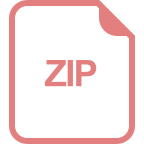
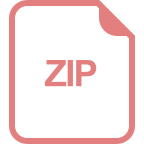
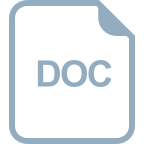
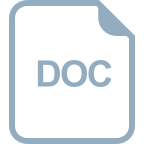