根据pt1000分度表,通过查表法得到pt1000的测量温度值,写出相应的函数代码,精确到误差不超过0.01 ,用结构体 c
时间: 2024-09-27 09:15:22 浏览: 65
在C语言中,为了实现基于PT1000分度表的温度测量并保证精度到0.01℃,我们可以创建一个结构体来存储PT1000数据,并编写一个函数来进行查询。首先,我们需要定义一个包含电阻值和对应温度的结构体。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdint.h>
// PT1000 温度-电阻值映射表的结构体
typedef struct {
float resistance; // 电阻值
float temperature; // 对应温度 (假设以摄氏度表示)
} Pt1000Resistance;
const Pt1000Resistance pt1000_table[] = {
{ 500.0f, 0.00 }, // 当电阻500欧姆时对应0℃
// ... 其他分度点的电阻值和温度依次填充 ...
{ 10000.0f, 100.0f } // 最高点,例如10000欧姆对应100℃
};
// 函数原型,输入电阻值,返回近似温度
float getTemperatureFromPt1000(float resistance);
// 查找最接近给定电阻值的分度点
float findClosestResistance(float resistance, const Pt1000Resistance table[], int size) {
float min_diff = INFINITY;
int closest_index = -1;
for (int i = 0; i < size; ++i) {
float diff = abs(resistance - table[i].resistance);
if (diff < min_diff) {
min_diff = diff;
closest_index = i;
}
}
return closest_index;
}
// 主函数获取温度并四舍五入到0.01℃
float getTemperatureFromPt1000(float resistance) {
int index = findClosestResistance(resistance, pt1000_table, sizeof(pt1000_table) / sizeof(pt1000_table[0]));
float temperature = pt1000_table[index].temperature;
// 考虑浮点数精度,可能会有微小偏差,所以四舍五入到0.01
return round(temperature * 100) / 100.0f;
}
int main() {
float measured_resistance = 600.0f; // 测量到的电阻值
float temperature = getTemperatureFromPt1000(measured_resistance);
printf("测得的温度约为 %.2f℃\n", temperature);
return 0;
}
```
阅读全文
相关推荐
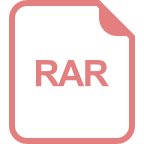
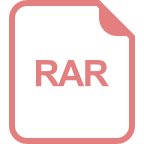

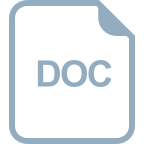
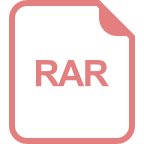
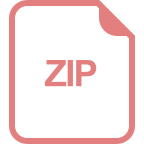
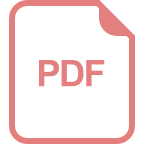
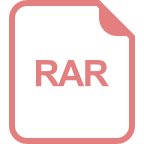
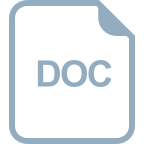






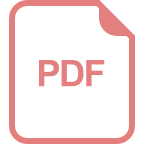
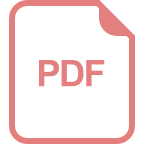