python 爬虫东方财富网融资融券
时间: 2023-10-11 08:06:28 浏览: 66
要爬取东方财富网的融资融券数据,可以按照以下步骤进行:
1. 首先,使用Python中的requests库发送GET请求获取股票列表页面的源代码。可以使用以下代码来实现:
```python
import requests
url = "http://quote.eastmoney.com/stock_list.html"
response = requests.get(url)
html = response.text
```
2. 然后,使用BeautifulSoup库解析源代码,提取出需要的股票代码和名称。可以使用以下代码来实现:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, "html.parser")
table = soup.find("div", class_="quotebody")
stocks = table.find_all("a")
for stock in stocks:
code = stock.text
if code.startswith("6"):
name = stock["target"]
print(f"股票代码:{code},股票名称:{name}")
```
3. 接下来,根据股票代码构造融资融券数据的URL,并发送GET请求获取数据。可以使用以下代码来实现:
```python
stock_code = "600000" # 这里以股票代码为600000的股票为例
url = f"http://nufm.dfcfw.com/EM_Finance2014NumericApplication/JS.aspx?type=CT&cmd={stock_code}2&sty=FCOIATA&st=z&sr=-1&p=1&ps=500&cb=jQuery18309439121673274709_1582166252397&_=1582166252402"
response = requests.get(url)
data = response.text
```
4. 最后,解析返回的数据,提取出需要的融资融券数据。可以使用以下代码来实现:
```python
import json
start_index = data.find("[")
end_index = data.rfind("]")
json_data = data[start_index:end_index+1]
parsed_data = json.loads(json_data)
for item in parsed_data:
date = item.split(",")[0].strip('"')
zdf = item.split(",")[2].strip('"')
rzye = item.split(",")[3].strip('"')
rzrqye = item.split(",")[4].strip('"')
print(f"日期:{date},涨跌幅:{zdf},融资余额:{rzye},融资融券余额:{rzrqye}")
```
相关推荐
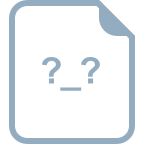
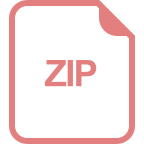














