帮我用python写一个pygame小游戏
时间: 2024-12-21 10:23:26 浏览: 3
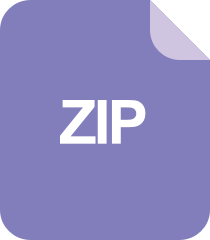
【新品爆款20个python小游戏】pygame小游戏全集,亲测源码可正常运行
在Python中,我们可以使用Pygame库来创建简单的2D游戏。以下是一个简单的例子,演示如何编写一个基础的井字棋(Tic Tac Toe)游戏:
```python
import pygame
import sys
# 初始化Pygame
pygame.init()
# 设置窗口大小和标题
screen_width = 600
screen_height = 400
win_title = "Tic Tac Toe"
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption(win_title)
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRID_COLOR = (150, 150, 150)
# 定义棋盘格子尺寸
grid_size = 75
gap = 10
# 创建9个方块
board = [[None] * 3 for _ in range(3)]
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
pos = pygame.mouse.get_pos()
row, col = pos // grid_size, pos // grid_size
if board[row][col] is None:
board[row][col] = 'X' if turn == 'O' else 'O'
turn = 'O' if turn == 'X' else 'X'
# 更新屏幕
screen.fill(BLACK)
for i in range(3):
for j in range(3):
pygame.draw.rect(screen, WHITE, (j * (grid_size + gap), i * (grid_size + gap), grid_size, grid_size))
text = board[i][j]
if text:
font = pygame.font.SysFont('Arial', 30)
text_surface = font.render(text, True, GRID_COLOR)
text_rect = text_surface.get_rect(center=(j * (grid_size + gap) + gap, i * (grid_size + gap) + gap))
screen.blit(text_surface, text_rect)
pygame.display.update()
# 游戏结束提示
if any(all(cell != None for cell in row) for row in board) or any(board[i][i] != None for i in range(3)) or any(board[i][2 - i] != None for i in range(3)):
winner = ''
for row in board:
if all(cell == 'X' for cell in row):
winner = 'X'
elif all(cell == 'O' for cell in row):
winner = 'O'
font = pygame.font.SysFont('Arial', 50)
message = "Player {} wins!".format(winner)
text_surface = font.render(message, True, WHITE)
text_rect = text_surface.get_rect(center=(screen_width // 2, screen_height // 2))
screen.fill(BLACK)
screen.blit(text_surface, text_rect)
pygame.display.update()
pygame.time.wait(3000) # 显示几秒再关闭窗口
pygame.quit()
```
这是一个基本版本,实际游戏中可能还需要添加更多的功能,如检测平局、玩家输入验证等。要运行这个游戏,你需要先安装Pygame库(`pip install pygame`),然后复制上述代码到`.py`文件中并运行。
阅读全文
相关推荐
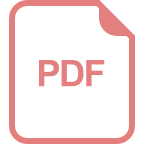
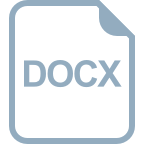
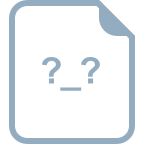
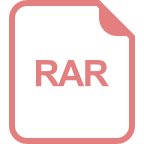
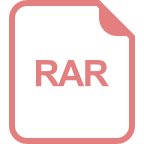
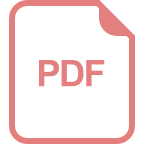
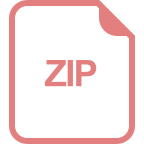
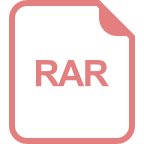
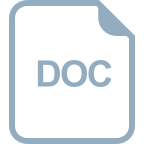




