遗传算法实现旅行商问题matlab
时间: 2023-10-14 07:07:41 浏览: 87
遗传算法可以用于解决旅行商问题(TSP)的优化。在matlab中使用遗传算法解决TSP问题的基本步骤如下:
1. 确定问题的目标:TSP问题的目标是找到一条最短路径,使得旅行商可以从一个城市出发,经过每个城市一次且仅一次,并回到起点城市。
2. 设计基因表示:将每个城市编码为一个基因,形成基因序列。基因序列的长度等于城市的数量。
3. 初始化种群:随机生成一定数量的个体作为初始种群。
4. 适应度计算:根据每个个体的基因序列计算其适应度,即路径的总长度。
5. 选择操作:使用选择算子从种群中选择一部分个体,作为下一代个体的父代。
6. 交叉操作:使用交叉算子将父代个体的基因进行交叉,生成新的子代个体。
7. 变异操作:使用变异算子对子代个体的基因进行变异,引入新的基因变体。
8. 更新种群:将父代和子代个体合并为新的种群。
9. 重复步骤4-8,直到达到停止条件(例如达到最大迭代次数或找到满意的解)。
10. 输出最优解:选择适应度最好的个体作为最优解。
相关问题
遗传算法解决旅行商问题 matlab
好的,遗传算法可以用来解决旅行商问题。以下是一个基于Matlab的遗传算法求解旅行商问题的简单示例代码:
```matlab
% 遗传算法解决旅行商问题示例代码
% 假设有6个城市,求解最短路径
% 城市坐标
city_pos = [0.5, 0.9; 0.3, 0.2; 0.8, 0.7; 0.1, 0.5; 0.7, 0.1; 0.6, 0.4];
% 生成初始种群
pop_size = 20; % 种群大小
pop = zeros(pop_size, 6); % 每个染色体表示一条路径,6个城市
for i = 1:pop_size
pop(i, :) = randperm(6);
end
% 遗传算法参数设置
max_gen = 50; % 最大迭代次数
pc = 0.8; % 交叉概率
pm = 0.1; % 变异概率
% 迭代
for gen = 1:max_gen
% 计算每个个体的适应度(路径长度)
fit = zeros(pop_size, 1);
for i = 1:pop_size
fit(i) = path_length(pop(i, :), city_pos);
end
% 选择
parents = selection(pop, fit);
% 交叉
offspring = crossover(parents, pc);
% 变异
offspring = mutation(offspring, pm);
% 合并父代和子代
pop = [parents; offspring];
% 精英保留
[best_fit, best_idx] = min(fit);
elite = pop(best_idx, :);
pop(end, :) = elite;
end
% 找到最优解
best_path = pop(best_idx, :);
best_length = path_length(best_path, city_pos);
disp(['最短路径长度:', num2str(best_length)]);
disp(['最短路径:', num2str(best_path)]);
```
其中,`path_length`函数用于计算路径长度,`selection`函数用于选择个体,`crossover`函数用于交叉,`mutation`函数用于变异。这些函数的具体实现方式可以根据实际情况进行调整。
遗传算法解决旅行商问题matlab
遗传算法可以用于解决旅行商问题,以下是一个使用MATLAB实现遗传算法解决旅行商问题的示例代码:
```matlab
% 定义旅行商问题的目标函数(适应度函数)
function fitness = tsp_fitness(population, distance_matrix)
[pop_size, ~] = size(population);
fitness = zeros(pop_size, 1);
for i = 1:pop_size
individual = population(i, :);
distance = 0;
for j = 1:numel(individual)-1
distance = distance + distance_matrix(individual(j), individual(j+1));
end
distance = distance + distance_matrix(individual(end), individual(1));
fitness(i) = 1/distance;
end
end
% 定义遗传算法的主函数
function best_path = tsp_ga(distance_matrix, num_cities, pop_size, num_generations)
% 生成初始种群
population = zeros(pop_size, num_cities);
for i = 1:pop_size
population(i, :) = randperm(num_cities);
end
best_fitness = zeros(num_generations, 1);
for gen = 1:num_generations
% 计算适应度值
fitness = tsp_fitness(population, distance_matrix);
% 选择操作(轮盘赌选择)
selected_indices = roulette_wheel_selection(fitness, pop_size);
selected_population = population(selected_indices, :);
% 交叉操作(部分映射交叉)
offspring_population = pmx_crossover(selected_population);
% 变异操作(交换变异)
mutated_population = swap_mutation(offspring_population);
% 更新种群
population = mutated_population;
% 记录每一代的最佳适应度值
best_fitness(gen) = max(fitness);
end
% 找到最佳路径
best_fitness_index = find(best_fitness == max(best_fitness));
best_path = population(best_fitness_index(1), :);
end
% 示例的距离矩阵
distance_matrix = [
0 3 2 4;
3 0 1 2;
2 1 0 3;
4 2 3 0
];
% 调用遗传算法函数
num_cities = size(distance_matrix, 1);
pop_size = 100;
num_generations = 1000;
best_path = tsp_ga(distance_matrix, num_cities, pop_size, num_generations);
disp('Best path:');
disp(best_path);
```
这段代码实现了遗传算法解决旅行商问题,包括目标函数的定义、遗传算法的主函数以及一些基本的遗传操作(选择、交叉、变异)。你可以根据自己的需求进行参数的调整和算法的扩展。希望对你有帮助!
阅读全文
相关推荐
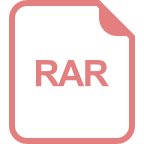
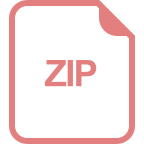
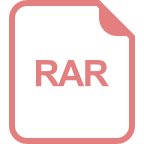
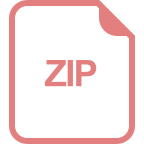
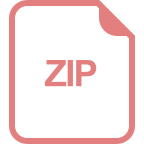
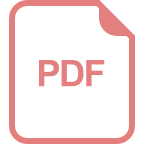
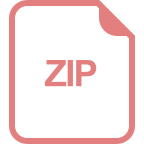
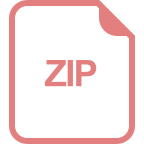
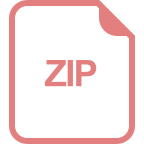
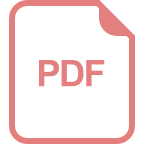
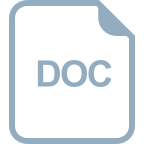
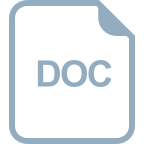
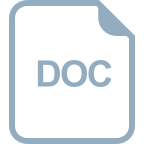