逐一统计文本文件中的字符数。 要求:统计某一文本文件中的大写字母、小写字母和数字出现的次数。
时间: 2024-12-19 16:27:56 浏览: 3
统计文本文件中的字符数通常需要逐行读取文件内容,并对每一行进行遍历分析。以下是Python的一个简单示例,用于统计大写字母、小写字母和数字的数量:
```python
def count_chars(filename):
with open(filename, 'r') as file:
content = file.read()
uppercase_count = sum(1 for char in content if char.isupper())
lowercase_count = sum(1 for char in content if char.islower())
digit_count = sum(1 for char in content if char.isdigit())
return uppercase_count, lowercase_count, digit_count
# 使用函数并打印结果
uppercase, lowercase, digits = count_chars('your_file.txt')
print(f"大写字母:{uppercase}")
print(f"小写字母:{lowercase}")
print(f"数字:{digits}")
相关问题
使用Python逐一统计文本文件的字符数,要求统一某一文本文件中的大写字母、小写字母和数字出现的次数
在Python中,你可以使用内置的`open()`函数打开文件,然后逐行读取内容,并对每一行进行字符计数。下面是一个简单的步骤来统计文本文件中的大写字母、小写字母和数字:
```python
def count_chars(file_path):
with open(file_path, 'r') as file:
content = file.read()
# 将字符串转为小写并移除非字母和数字的字符
cleaned_content = ''.join(c.lower() for c in content if c.isalnum())
# 初始化计数器
uppercase_count = lowercase_count = digit_count = 0
# 统计每个字符类型的数量
for char in cleaned_content:
if char.isupper():
uppercase_count += 1
elif char.islower():
lowercase_count += 1
else:
digit_count += 1
return uppercase_count, lowercase_count, digit_count
# 使用函数并打印结果
file_name = "your_text_file.txt" # 替换为你需要处理的文件名
uppercase, lowercase, digits = count_chars(file_name)
print(f"Uppercase letters: {uppercase}")
print(f"Lowercase letters: {lowercase}")
print(f"Digits: {digits}")
任务:逐一统计文本文件中的字符数:统计文本文件中的大写字母、小写字母和数字出现的次数 提示: (1)读取文件内容后,使用字符串内置方法isupper()、islower()、isdigit()判断字符类别 (2)打开文件时,未指定打开模式,默认为r模式,编码方式为UTF-8,可以写成 file = open(“src.text”,'r',encoding='utf-8') (3)通过循环逐一判断文件中的每一个字符是否是大写字母、小写字母或数字。 拓展内容: 将统计后的数据以如下格式,写入到dest.csv文件中 字符类型 数量 大写 20 小写 15 数字 50
好的,我已经明白了你的问题。以下是 Python 代码实现,可以逐一统计文本文件中的字符数并将结果写入到 dest.csv 文件中:
```python
# 打开文件
with open('src.txt', 'r', encoding='utf-8') as f:
content = f.read()
# 初始化计数器
upper_count = 0
lower_count = 0
digit_count = 0
# 统计字符数
for char in content:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
# 写入结果到 dest.csv 文件中
with open('dest.csv', 'w', encoding='utf-8') as f:
f.write('字符类型,数量\n')
f.write('大写,{0}\n'.format(upper_count))
f.write('小写,{0}\n'.format(lower_count))
f.write('数字,{0}\n'.format(digit_count))
```
注意:这段代码中的 `src.txt` 文件名需要替换成你要统计字符数的文件名。`dest.csv` 文件是写入结果的文件名,如果该文件不存在,程序将会自动创建。
阅读全文
相关推荐
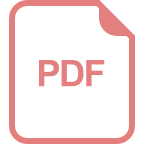
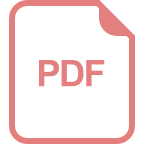
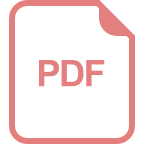
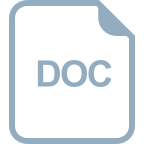
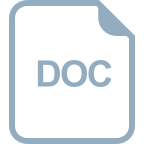










