如何在Python中使用dbutils.pooled_db模块连接MySQL 8.0数据库并配置auth_plugin_map为'mysql_native_password'?
时间: 2024-11-09 20:19:31 浏览: 48
要使用`dbutils.pooled_db`模块连接MySQL 8.0数据库并配置`auth_plugin_map`为`mysql_native_password`,首先确保已经安装了`pymysql`库。然后,你可以按照以下步骤进行:
1. **导入所需模块**:
```python
from dbutils.pooled_db import PooledDB
```
2. **设置连接参数**:
- `host`: 数据库服务器地址
- `user`: 用户名
- `passwd`: 密码(这里以哈希形式存储或安全方式传递)
- `db`: 要连接的数据库名称
- `port`: 数据库端口,默认为3306
- `pool_size`: 连接池大小,可选参数
- `max_overflow`: 池溢出时允许额外的连接数,可选参数
- `auth_plugin_map`: 自定义认证插件映射,这里是`{'mysql_native_password': 'caching_sha2_password'}`,因为`mysql_native_password`已不再安全,但在这里作为示例。
3. **创建连接池**:
```python
auth_plugin_map = {'mysql_native_password': 'caching_sha2_password'}
params = {
'host': 'localhost',
'user': 'root',
'password': 'your_hashed_password', # 以哈希形式存储的密码
'database': 'Mysql8',
'port': 3306,
'auth_plugin_map': auth_plugin_map,
}
pool = PooledDB(
creator=pymysql, # 使用pymysql创建连接
maxconnections=10, # 连接池大小
mincached=5, # 最小空闲连接数
maxcached=20, # 最大空闲连接数
maxshared=3, # 最大共享连接数
blocking=True, # 阻塞等待连接
maxusage=None, # 连接最大重用次数
setsession=[], # 设置会话选项的列表
ping=0, # 是否启用ping检测,0表示不检测
**params
)
```
4. **从池中获取连接**:
```python
connection = pool.connection()
```
5. **执行SQL语句**:
```python
cursor = connection.cursor()
# 执行查询或其他操作
```
6. **关闭连接**:
```python
cursor.close()
connection.close()
```
7. **错误处理**:
如果在操作过程中遇到异常,可以捕获并处理,如示例[2]所示:
```python
try:
with pool.connection() as conn:
# ... 执行SQL语句 ...
except Exception as e:
print(f"Error occurred: {e}")
```
记得替换`'your_hashed_password'`为你实际的哈希密码。
阅读全文
相关推荐
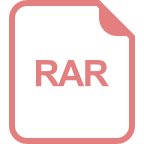
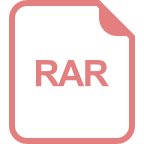
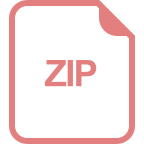









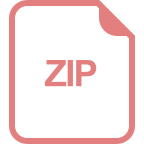




