aes_ccm算法代码
时间: 2023-07-24 21:02:26 浏览: 372
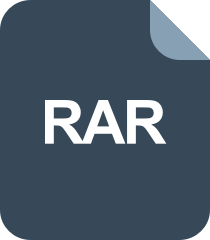
AES算法代码
### 回答1:
AES-CCM算法是一种对称加密算法,用于对数据进行机密性和完整性的保护。它结合了AES加密和CCM模式,并提供了一种高效的方式来进行安全通信。
下面是AES-CCM算法的代码实现:
```
import hashlib
from Crypto.Cipher import AES
from Crypto.Util import Counter
# 定义函数,用于实现AES-CCM算法加密
def aes_ccm_encrypt(key, nonce, plaintext, associated_data):
# 设置加密模式为CTR
ctr = Counter.new(128, initial_value=int.from_bytes(nonce, byteorder='big'))
aes = AES.new(key, AES.MODE_CTR, counter=ctr)
# 计算指定数据的MAC值
mac_key = hashlib.sha256(bytes.fromhex(key)).digest()
aes_mac = AES.new(mac_key, AES.MODE_ECB)
mac = aes_mac.encrypt(associated_data)
# 对明文进行加密
ciphertext = aes.encrypt(plaintext)
# 将MAC值与密文进行合并
encrypted_data = mac + ciphertext
return encrypted_data
# 定义函数,用于实现AES-CCM算法解密
def aes_ccm_decrypt(key, nonce, encrypted_data, associated_data):
# 设置加密模式为CTR
ctr = Counter.new(128, initial_value=int.from_bytes(nonce, byteorder='big'))
aes = AES.new(key, AES.MODE_CTR, counter=ctr)
# 计算指定数据的MAC值
mac_key = hashlib.sha256(bytes.fromhex(key)).digest()
aes_mac = AES.new(mac_key, AES.MODE_ECB)
mac = aes_mac.encrypt(associated_data)
# 将MAC值与密文分离
received_mac = encrypted_data[:16]
ciphertext = encrypted_data[16:]
# 验证MAC值是否一致
if mac != received_mac:
raise ValueError("MAC verification failed")
# 对密文进行解密
plaintext = aes.decrypt(ciphertext)
return plaintext
# 测试示例
key = '2b7e151628aed2a6abf7158809cf4f3c'
nonce = '000000300000000000000000'
plaintext = 'Hello, World!'
associated_data = 'Test'
encrypted_data = aes_ccm_encrypt(bytes.fromhex(key), bytes.fromhex(nonce), plaintext.encode(), associated_data.encode())
decrypted_data = aes_ccm_decrypt(bytes.fromhex(key), bytes.fromhex(nonce), encrypted_data, associated_data.encode())
print("加密后的数据:", encrypted_data.hex())
print("解密后的数据:", decrypted_data.decode())
```
以上代码实现了AES-CCM算法的加密和解密过程。可以通过指定密钥、随机数、明文和关联数据进行加密,然后再通过指定密钥、随机数、加密后的数据和关联数据进行解密。最后打印出加密后的数据和解密后的数据。
### 回答2:
AES-CCM算法是一种以AES加密和CCM(Counter with CBC-MAC)模式结合的加密算法。这个算法主要用于提供对称加密以及完整性和认证的安全保证。
AES-CCM算法的实现可以使用一些编程语言来进行。以下是一个Python语言的代码示例:
```
from Crypto.Cipher import AES
from Crypto.Util import Counter
def aes_ccm_encrypt(key, nonce, plaintext, mac_len, auth_data):
counter = Counter.new(64, prefix=nonce)
cipher = AES.new(key, AES.MODE_CTR, counter=counter)
ciphertext = cipher.encrypt(plaintext)
ccm_cipher = AES.new(key, AES.MODE_CBC, nonce)
mac = ccm_cipher.encrypt(ciphertext[-auth_data:])
return nonce + ciphertext + mac
def aes_ccm_decrypt(key, ciphertext, mac_len, auth_data):
nonce = ciphertext[:16]
ciphertext = ciphertext[16:-mac_len]
mac = ciphertext[-mac_len:]
ccm_cipher = AES.new(key, AES.MODE_CBC, nonce)
expected_mac = ccm_cipher.encrypt(ciphertext[-auth_data:])
if mac != expected_mac:
raise ValueError("Message authentication failed!")
counter = Counter.new(64, prefix=nonce)
cipher = AES.new(key, AES.MODE_CTR, counter=counter)
plaintext = cipher.decrypt(ciphertext)
return plaintext
# 以下为使用示例
key = b'Sixteen byte key'
nonce = b'Initialization V'
plaintext = b'Plain text'
mac_len = 8
auth_data = b'Additional data'
ciphertext = aes_ccm_encrypt(key, nonce, plaintext, mac_len, auth_data)
decrypted_plaintext = aes_ccm_decrypt(key, ciphertext, mac_len, auth_data)
print("Ciphertext:", ciphertext)
print("Decrypted plaintext:", decrypted_plaintext)
```
以上代码演示了如何使用AES-CCM算法进行加密和解密操作。其中,`key`是16字节长度的加密密钥,`nonce`是12字节长度的随机值,`plaintext`是待加密的明文数据,`mac_len`是MAC(Message Authentication Code)的长度,`auth_data`是附加数据。加密函数`aes_ccm_encrypt`将明文加密成密文,解密函数`aes_ccm_decrypt`将密文解密成明文。最后的输出显示了密文和解密后的明文。
注意,代码中使用了`Crypto`库来实现AES加密和CCM模式操作,需要提前安装这个库。
阅读全文
相关推荐
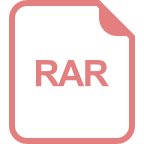
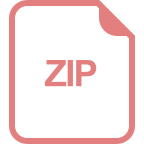
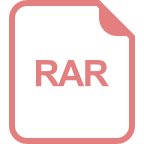

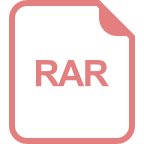
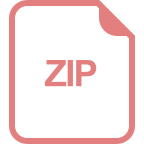
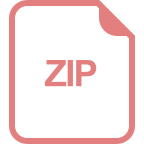
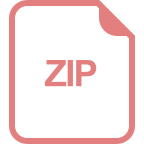
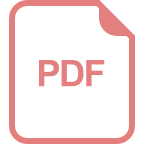
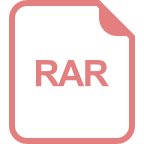
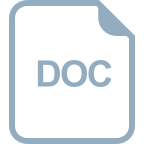
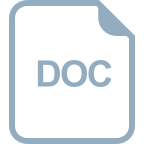
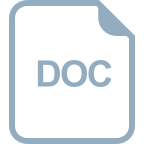
