while生成字母表
时间: 2024-09-22 08:04:24 浏览: 34
"while"通常用于循环结构,在某些编程语言中(如Python),可以结合生成器(generator)或列表推导式(list comprehension)来动态生成字母表。下面是一个简单的例子:
如果你想生成英文小写字母表,你可以这样做:
```python
import string
def generate_alphabet(start=string.ascii_lowercase[0]):
letter = start
while True:
yield letter
letter = chr(ord(letter) + 1) # 循环递增ASCII码值
if letter > 'z': # 当达到'z'时,跳回'a'
letter = 'a'
# 使用生成器
for char in generate_alphabet():
print(char)
```
这个函数会无限循环生成小写字母表,直到你停止迭代。如果你想在某个固定长度内生成,可以在`for`循环中设置一个限制。
相关问题
c语言随机生成字母链表,C语言实现链表贪吃蛇
生成字母链表可以使用C语言的随机数生成函数rand(),然后将生成的数字转换成字母即可。以下是生成长度为n的字母链表的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
typedef struct Node {
char data;
struct Node *next;
} Node;
Node *createList(int n) {
Node *head = NULL;
Node *tail = NULL;
srand(time(NULL));
for (int i = 0; i < n; i++) {
Node *node = (Node *) malloc(sizeof(Node));
node->data = rand() % 26 + 'a';
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
void printList(Node *head) {
while (head != NULL) {
printf("%c ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
Node *list = createList(10);
printList(list);
return 0;
}
```
接下来是实现链表贪吃蛇的示例代码。链表贪吃蛇可以通过一个链表来表示蛇的身体,每个节点表示一个身体部位,头节点表示蛇头,尾节点表示蛇尾。蛇的运动可以通过在链表头部添加一个节点来实现。以下是示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 30
#define HEIGHT 20
typedef struct Point {
int x;
int y;
} Point;
typedef struct Node {
Point data;
struct Node *next;
} Node;
typedef enum Direction {
UP, DOWN, LEFT, RIGHT
} Direction;
Node *createSnake(Point head) {
Node *node = (Node *) malloc(sizeof(Node));
node->data = head;
node->next = NULL;
return node;
}
void printSnake(Node *snake) {
while (snake != NULL) {
printf("(%d,%d) ", snake->data.x, snake->data.y);
snake = snake->next;
}
printf("\n");
}
void drawMap(Node *snake, Point food) {
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (i == 0 || i == HEIGHT - 1 || j == 0 || j == WIDTH - 1) {
printf("#");
} else if (i == food.y && j == food.x) {
printf("$");
} else {
int found = 0;
Node *p = snake;
while (p != NULL) {
if (p->data.x == j && p->data.y == i) {
printf("*");
found = 1;
break;
}
p = p->next;
}
if (!found) {
printf(" ");
}
}
}
printf("\n");
}
}
int isCollided(Node *snake) {
Node *p = snake->next;
while (p != NULL) {
if (p->data.x == snake->data.x && p->data.y == snake->data.y) {
return 1;
}
p = p->next;
}
return 0;
}
int isInMap(Point point) {
return point.x > 0 && point.x < WIDTH - 1 && point.y > 0 && point.y < HEIGHT - 1;
}
Point generateFood(Node *snake) {
Point food;
do {
food.x = rand() % (WIDTH - 2) + 1;
food.y = rand() % (HEIGHT - 2) + 1;
} while (!isInMap(food) || isCollided(snake));
return food;
}
void moveSnake(Node **snake, Direction direction, Point food, int *score) {
Point head = (*snake)->data;
switch (direction) {
case UP:
head.y--;
break;
case DOWN:
head.y++;
break;
case LEFT:
head.x--;
break;
case RIGHT:
head.x++;
break;
}
Node *node = (Node *) malloc(sizeof(Node));
node->data = head;
node->next = *snake;
*snake = node;
if (head.x == food.x && head.y == food.y) {
food = generateFood(*snake);
*score += 10;
} else {
Node *tail = *snake;
while (tail->next->next != NULL) {
tail = tail->next;
}
free(tail->next);
tail->next = NULL;
}
drawMap(*snake, food);
}
int main() {
srand(time(NULL));
Point head = {WIDTH / 2, HEIGHT / 2};
Node *snake = createSnake(head);
Direction direction = RIGHT;
Point food = generateFood(snake);
int score = 0;
while (1) {
if (_kbhit()) {
char ch = _getch();
if (ch == 'w' && direction != DOWN) {
direction = UP;
} else if (ch == 's' && direction != UP) {
direction = DOWN;
} else if (ch == 'a' && direction != RIGHT) {
direction = LEFT;
} else if (ch == 'd' && direction != LEFT) {
direction = RIGHT;
}
}
moveSnake(&snake, direction, food, &score);
if (!isInMap(snake->data) || isCollided(snake)) {
printf("Game Over! Score: %d\n", score);
break;
}
Sleep(100);
}
return 0;
}
```
代码中使用了Windows系统的控制台函数和键盘输入函数,需要在Windows系统下运行。
生成一个词法分析器包括字母表 构词规则(正规式 状态转化矩阵 产生式)用c++
当然可以!下面是一个简单的词法分析器的实现示例,使用C++编写:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <map>
using namespace std;
// 词法分析器类
class LexicalAnalyzer {
private:
vector<string> tokenNames; // Token类型名称列表
map<string, string> regexMap; // 正则表达式映射
map<string, vector<string>> transitions; // 状态转移矩阵
public:
// 构造函数,初始化字母表、构词规则和状态转移矩阵
LexicalAnalyzer() {
tokenNames = {"IDENTIFIER", "NUMBER", "OPERATOR", "KEYWORD"};
regexMap = {
{"IDENTIFIER", "[a-zA-Z][a-zA-Z0-9]*"},
{"NUMBER", "\\d+"},
{"OPERATOR", "[+\\-*/]"},
{"KEYWORD", "(if|else|while)"}
};
transitions = {
{"START", {"IDENTIFIER", "NUMBER", "OPERATOR", "KEYWORD"}},
{"IDENTIFIER", {"IDENTIFIER", "NUMBER"}},
{"NUMBER", {"NUMBER"}},
{"OPERATOR", {}},
{"KEYWORD", {}}
};
}
// 执行词法分析
void analyze(string input) {
int pos = 0;
string state = "START";
while (pos < input.length()) {
string currentChar = string(1, input[pos]);
string nextState = getNextState(state, currentChar);
if (nextState == "") {
// 当前状态无法转移到下一个状态,输出当前Token并返回START状态
string tokenType = getTokenType(state);
cout << tokenType << ": " << input.substr(0, pos) << endl;
state = "START";
} else {
// 当前状态可以转移到下一个状态,继续处理下一个字符
state = nextState;
pos++;
}
}
// 处理最后一个Token
if (state != "START") {
string tokenType = getTokenType(state);
cout << tokenType << ": " << input.substr(0, pos) << endl;
}
}
private:
// 获取下一个状态
string getNextState(string currentState, string inputChar) {
if (transitions.find(currentState) != transitions.end()) {
vector<string> possibleStates = transitions[currentState];
for (string nextState : possibleStates) {
string regexPattern = regexMap[nextState];
if (inputChar == " " || regexPattern == "" || regex_match(inputChar, regex(regexPattern))) {
return nextState;
}
}
}
return "";
}
// 获取Token类型名称
string getTokenType(string state) {
for (string tokenName : tokenNames) {
if (transitions.find(tokenName) != transitions.end() && transitions[tokenName].empty()) {
if (state == tokenName) {
return tokenName;
}
}
}
return "";
}
};
// 测试
int main() {
LexicalAnalyzer lexer;
string input = "int x = 10 + y; if(x > 5) { y = y + 1; }";
lexer.analyze(input);
return 0;
}
```
这个例子实现了一个简单的词法分析器,可以识别标识符、数字、运算符和关键字。你可以根据需要添加更多的正则表达式和状态转移规则。运行上述代码将输出词法分析结果。
希望这个例子能帮助你理解如何使用C++实现词法分析器。如果有任何问题,请随时提问!
阅读全文
相关推荐
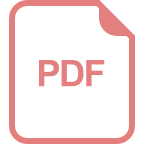
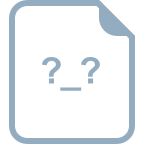
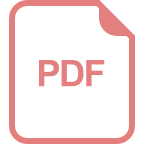
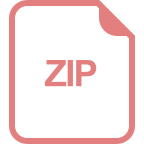
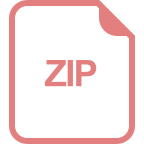
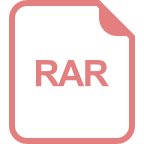
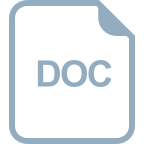









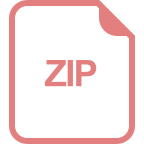