RC4加解密源码(C语言描述)
时间: 2024-11-03 22:17:55 浏览: 3
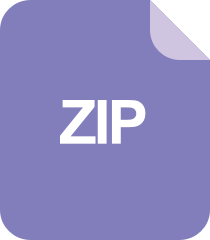
c代码-C语言版RC4算法 加解密
RC4是一种流密码算法,常用于对网络数据进行实时加密。下面是C语言的一个简化版RC4加解密源码概述:
```c
#include <stdio.h>
#include <stdlib.h>
// 初始化S盒数组
void init_key(char *key, int key_len) {
unsigned char key_schedule[key_len + 256];
int j = 0, x = 0;
for (int i = 0; i < 256; ++i) {
key_schedule[i] = i;
}
for (int i = 0; i < key_len; ++i) {
x = (x + key_schedule[i] + key[i]) % 256;
swap(&key_schedule[i], &key_schedule[x]);
}
// 将剩余部分复制到前256位
for (j = key_len; j < 256; ++j) {
x = (x + 1) % 256;
swap(&key_schedule[j], &key_schedule[x]);
}
}
// 执行一次轮函数
void shift_row(unsigned char *state) {
unsigned char temp = state[0];
state[0] = state[4];
state[4] = state[8];
state[8] = state[12];
state[12] = temp;
}
// 执行一次混洗
void mix_column(unsigned char *column) {
static const unsigned char sbox[] = {
0x0, 0x3, 0x6, 0x9, 0xc, 0xf, 0xa, 0xd,
0xe, 0xb, 0x8, 0x5, 0x2, 0x1, 0x7, 0x4
};
unsigned char t = column[0];
for (int i = 0; i < 4; ++i) {
column[i] = column[i] ^ sbox[(t >> 5) & 0xf];
t <<= 1;
if (t >= 0x100)
t ^= 0x11b;
}
column[4] = t;
}
// 加密和解密通用函数
void rc4_encrypt_or_decrypt(char *input, char *output, char *key, int len, int is_encrypt) {
init_key(key, strlen(key));
unsigned char state[256];
for (int i = 0; i < len; ++i) {
x = (x + 1) % 256;
y = (y + state[x]) % 256;
swap(&state[x], &state[y]);
if (is_encrypt)
output[i] = input[i] ^ state[(state[x] + state[y]) % 256];
else
output[i] = input[i] ^ state[state[(state[x] + state[y]) % 256]];
}
}
int main() {
char key[] = "example"; // 示例密钥
char plaintext[] = "Hello, World!";
char ciphertext[sizeof(plaintext)];
// 加密
rc4_encrypt_or_decrypt(plaintext, ciphertext, key, sizeof(plaintext), 1);
printf("Encrypted text: %s\n", ciphertext);
// 解密
rc4_encrypt_or_decrypt(ciphertext, ciphertext, key, sizeof(plaintext), 0);
printf("Decrypted text: %s\n", ciphertext);
return 0;
}
```
这个示例展示了如何创建一个简单的RC4加密和解密函数,实际应用中可能会有更复杂的错误处理和性能优化。请注意,RC4已经被认为不够安全,不适合现代加密需求。
阅读全文
相关推荐
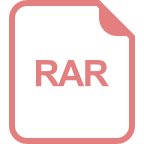
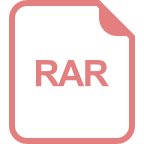
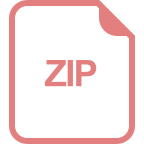
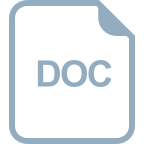
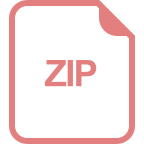
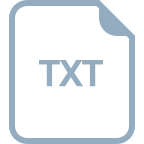
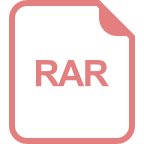
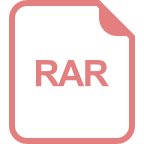
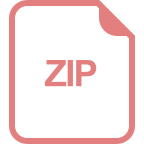
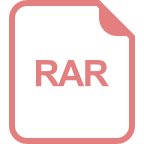
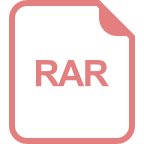
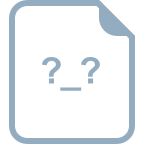
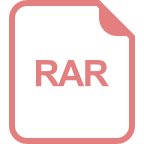
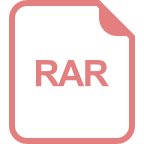
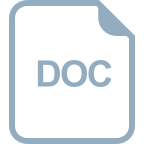
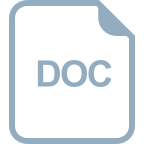
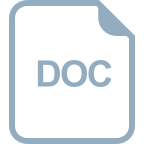
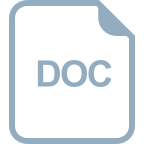