编写python代码 使得根据WGS84的经纬度坐标和高程以及卫星影像的RPC参数计算得到相应的像素坐标
时间: 2024-10-09 10:01:36 浏览: 62
在Python中,要根据WGS84坐标、高程和卫星影像的RPC (Rectified Pixel Coordinates) 参数来计算出对应的像素坐标,你可以使用一些开源库如`pyproj`, `rasterio` 或者 `gdal` 等。这里是一个简化的步骤:
首先,安装必要的库:
```bash
pip install pyproj rasterio
```
然后,假设你已经有了WGS84坐标(lon, lat, height)、投影信息(如EPSG code),以及RPC参数(包括xllcorner, yllcorner, cellsize等)。以下是一个示例代码片段:
```python
import numpy as np
from pyproj import Proj, transform
# 假设已知数据
wgs84_proj = Proj(proj='latlong', ellps='WGS84')
rpc_proj = Proj(init='epsg:3857') # 假设是Web Mercator投影
wgs84_coords = (lon, lat, height)
rpc_params = {'xllcorner': xllcorner, 'yllcorner': yllcorner, 'cellsize': cellsize}
def wgs84_to_rpc(wgs84_point, rpc_params):
# 将三维坐标转换为二维平面坐标
earth_radius_meters = 6371000 # WGS84平均半径,米
lat_rad = np.radians(wgs84_point[1])
height_above_ellipsoid = wgs84_point[2]
# 高程修正(如果需要)
orthometric_height = height_above_ellipsoid + earth_radius_meters * np.tan(lat_rad)
# 转换为直角坐标系
x, y = transform(wgs84_proj, rpc_proj, wgs84_point[0], orthometric_height)
# RPC参数调整像素坐标
pixel_x = (x - rpc_params['xllcorner']) / rpc_params['cellsize']
pixel_y = (y - rpc_params['yllcorner']) / rpc_params['cellsize']
return pixel_x, pixel_y
pixel_coords = wgs84_to_rpc(wgs84_coords, rpc_params)
print(f"像素坐标为: ({pixel_coords[0]}, {pixel_coords[1]})")
阅读全文
相关推荐
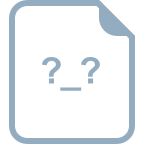
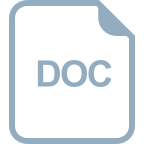
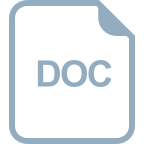
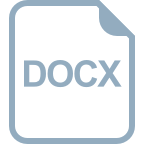
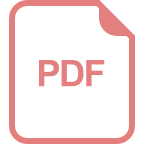
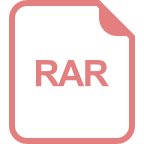
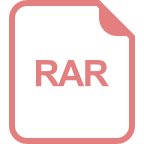
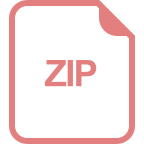
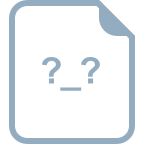
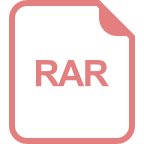
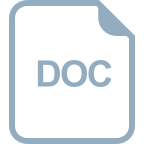