用Python计算附和导线测量
时间: 2024-05-24 12:07:58 浏览: 174
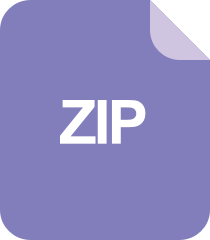
c#附和导线平差 使用vb+excel的方式进行导线测量平差的程序的设计vb负责全部的计算与交互
附和导线测量是一种常见的地形测量方法,主要用于获取两个点之间的距离和方位角。在Python中,可以使用数学库和科学计算库来实现这个过程。具体实现方式如下:
1. 计算两个点的经纬度坐标差值,得到距离值
2. 计算两个点的方位角,即两个点之间的夹角
3. 将方位角转化为正北方向的角度
4. 使用三角函数计算出相邻边和斜边的比值,得到正切值
5. 根据正切值和距离值计算出相邻边和斜边的长度
以下是一个示例代码,其中输入的点的经纬度坐标以及距离单位需要自行定义:
```
import math
def calc_distance(lat1, lon1, lat2, lon2):
earth_radius = 6378.137 # 地球半径
rad_lat1 = math.radians(lat1)
rad_lat2 = math.radians(lat2)
a = rad_lat1 - rad_lat2
b = math.radians(lon1) - math.radians(lon2)
s = 2 * math.asin(math.sqrt(pow(math.sin(a/2),2) + math.cos(rad_lat1)*math.cos(rad_lat2)*pow(math.sin(b/2),2)))
s *= earth_radius
return s
def calc_azimuth(lat1, lon1, lat2, lon2):
rad_lat1 = math.radians(lat1)
rad_lat2 = math.radians(lat2)
a = rad_lat1 - rad_lat2
b = math.radians(lon1) - math.radians(lon2)
angle = math.atan2(math.sin(b)*math.cos(rad_lat2), math.cos(rad_lat1)*math.sin(rad_lat2) - math.sin(rad_lat1)*math.cos(rad_lat2)*math.cos(b))
angle = math.degrees(angle)
if angle < 0:
angle += 360
return angle
lat1 = 39.90872
lon1 = 116.39748
lat2 = 39.90869
lon2 = 116.39748
distance = calc_distance(lat1, lon1, lat2, lon2)
azimuth = calc_azimuth(lat1, lon1, lat2, lon2)
azimuth_north = (450 - azimuth) % 360 # 将方位角转化为正北方向的角度
tan_value = math.tan(math.radians(azimuth_north))
adjacent_edge = distance / (math.sqrt(1 + pow(tan_value, 2)))
oblique_edge = adjacent_edge * tan_value
print("距离:%.4f 米" % distance)
print("方位角:%.4f 度" % azimuth)
print("正北方向角度:%.4f 度" % azimuth_north)
print("相邻边长度:%.4f 米" % adjacent_edge)
print("斜边长度:%.4f 米" % oblique_edge)
```
阅读全文
相关推荐
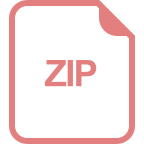
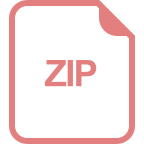
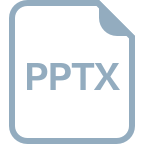
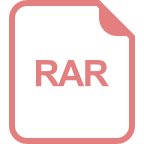
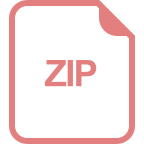
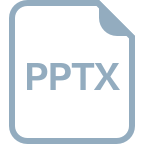
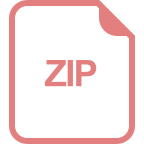
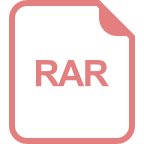
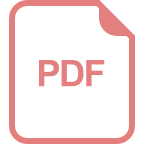
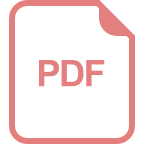
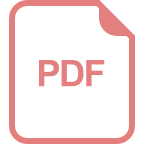
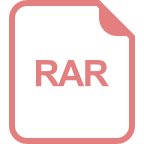
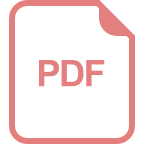