输入一串字符(字符串长度不超过200),从中挑选出小写字母a-z,其余字符剔除,并对挑选出的字符进行a-z的排序,输出排序后的字符串,如果没有挑选出任何有效字符,则输出error。
时间: 2024-04-16 07:28:24 浏览: 13
你可以使用以下的 C 代码来解决这个问题:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void sortString(char *str) {
int len = strlen(str);
char sortedStr[27]; // 26个小写字母加上结尾的'\0'
int count = 0; // 记录挑选出的字符数量
// 挑选出小写字母并存储到sortedStr数组中
for (int i = 0; i < len; i++) {
if (islower(str[i])) {
sortedStr[count] = str[i];
count++;
}
}
// 如果没有挑选出任何有效字符,则输出error
if (count == 0) {
printf("error\n");
return;
}
// 对挑选出的字符进行排序
for (int i = 0; i < count - 1; i++) {
for (int j = i + 1; j < count; j++) {
if (sortedStr[i] > sortedStr[j]) {
char temp = sortedStr[i];
sortedStr[i] = sortedStr[j];
sortedStr[j] = temp;
}
}
}
// 输出排序后的字符串
for (int i = 0; i < count; i++) {
printf("%c", sortedStr[i]);
}
printf("\n");
}
int main() {
char str[201];
printf("请输入一串字符:");
scanf("%200s", str);
sortString(str);
return 0;
}
```
你可以在输入一串字符后,程序将挑选出小写字母并对其进行排序,然后输出排序后的字符串。如果没有挑选出任何有效字符,则输出"error"。
相关推荐
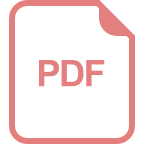
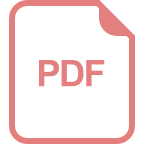
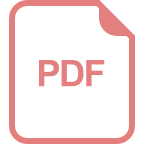







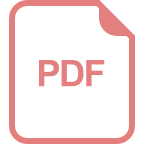
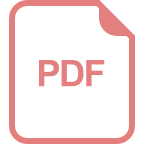
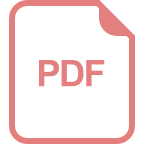
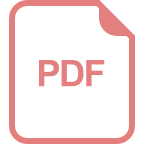
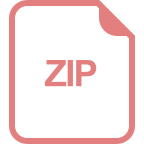
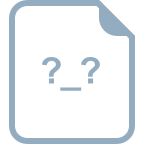
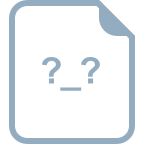