用c语言编写程序实现链表的创建,链表的输出,链表中查找数据。创建链表 输出链表、查找功能分别用函数实现。
时间: 2025-01-07 21:00:46 浏览: 2
在C语言中,我们可以使用结构体(struct)来表示链表节点,并定义相应的函数来操作链表。以下是简单的链表实现步骤:
1. **定义链表节点**:
```c
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. **创建链表**(链表头结点通常为空):
```c
Node* createList() {
Node* head = NULL;
return head;
}
```
3. **添加节点到链表**:
```c
void insert(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
newNode->data = value;
newNode->next = *head; // 新节点指向当前头部
*head = newNode; // 更新头部
}
```
4. **遍历并输出链表**:
```c
void printList(Node* head) {
if (head == NULL) {
printf("链表为空\n");
} else {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
}
```
5. **查找特定值**:
```c
Node* search(Node* head, int value) {
Node* current = head;
while (current != NULL) {
if (current->data == value) {
return current;
}
current = current->next;
}
return NULL; // 如果未找到,则返回NULL
}
```
要使用上述函数,你可以先创建链表,然后插入一些元素,最后打印链表并查找指定值。例如:
```c
int main() {
Node* listHead = createList();
insert(&listHead, 10);
insert(&listHead, 20);
insert(&listHead, 30);
printList(listHead); // 输出: 10 -> 20 -> 30 -> NULL
Node* foundNode = search(listHead, 20);
if (foundNode) {
printf("找到值 %d\n", foundNode->data);
} else {
printf("没找到该值\n");
}
return 0;
}
```
阅读全文
相关推荐




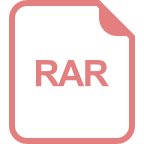













