java代码中svngit svn 如何判断目录是否存在
时间: 2024-05-01 14:15:35 浏览: 117
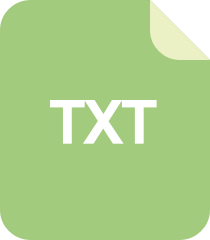
根据路径判断指定目录是否存在
在Java代码中,可以使用SVNKit或JGit库来操作SVN和Git版本控制系统。下面是使用SVNKit和JGit判断目录是否存在的示例代码:
使用SVNKit判断SVN目录是否存在的代码示例:
```java
import org.tmatesoft.svn.core.SVNException;
import org.tmatesoft.svn.core.SVNURL;
import org.tmatesoft.svn.core.internal.io.dav.DAVRepositoryFactory;
import org.tmatesoft.svn.core.wc.SVNClientManager;
public class SVNExample {
public static void main(String[] args) {
// 初始化SVN库
DAVRepositoryFactory.setup();
String svnUrl = "svn://example.com/svn/repository";
String svnDirectory = "/path/to/directory";
try {
SVNURL url = SVNURL.parseURIEncoded(svnUrl);
SVNClientManager clientManager = SVNClientManager.newInstance();
boolean directoryExists = clientManager.getWCClient().doInfo(url.appendPath(svnDirectory, false), null).getKind().isDirectory();
if (directoryExists) {
System.out.println("The directory exists.");
} else {
System.out.println("The directory does not exist.");
}
} catch (SVNException e) {
e.printStackTrace();
}
}
}
```
使用JGit判断Git目录是否存在的代码示例:
```java
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import org.eclipse.jgit.lib.Repository;
import org.eclipse.jgit.storage.file.FileRepositoryBuilder;
import java.io.File;
import java.io.IOException;
public class GitExample {
public static void main(String[] args) {
String gitDirectory = "/path/to/repository";
try {
Repository repository = FileRepositoryBuilder.create(new File(gitDirectory));
Git git = new Git(repository);
boolean directoryExists = repository.getDirectory().exists();
if (directoryExists) {
System.out.println("The directory exists.");
} else {
System.out.println("The directory does not exist.");
}
} catch (IOException | GitAPIException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
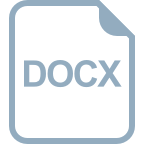
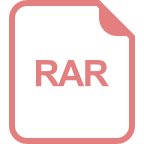
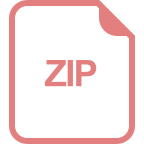
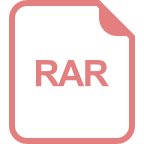
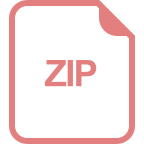
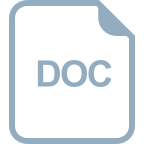
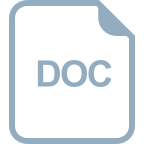
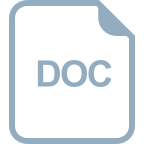
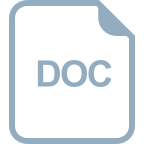
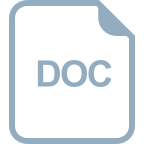
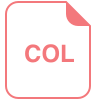
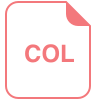
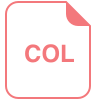
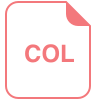
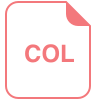
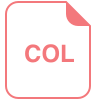
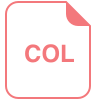