用C语言编写此实验代码
时间: 2024-11-20 17:36:01 浏览: 12
编写这个实验的C语言代码主要包括以下几个部分:
```c
#include <stdio.h>
#include <string.h>
// 定义字母映射表
const char encrypt_table[] = "n g z q t c o b m u h e l k p d a w x f y i v r s j";
const char decrypt_table[] = "a b c d e f g h i j k l m n o p q r s t u v w x y z n";
// 加密函数
char encrypt(char ch) {
for (int i = 0; i < strlen(encrypt_table); ++i) {
if (ch == encrypt_table[i]) {
return decrypt_table[i];
}
}
// 如果找不到映射,返回原字符(这里仅作示例,实际可能需要处理)
return ch;
}
// 解密函数
char decrypt(char ch) {
for (int i = 0; i < strlen(decrypt_table); ++i) {
if (ch == decrypt_table[i]) {
return encrypt_table[i];
}
}
return ch;
}
int main() {
char input[100]; // 存储用户输入
printf("请输入您的学号姓名(只接受英文字符):");
fgets(input, sizeof(input), stdin);
// 移除fgets读入的换行符
size_t len = strlen(input);
if (input[len - 1] == '\n') {
input[len - 1] = '\0';
}
// 加密字符串
char encrypted[100];
strcpy(encrypted, input);
for (size_t i = 0; encrypted[i]; ++i) {
encrypted[i] = encrypt(encrypted[i]);
}
// 输出加密后的字符串
printf("加密后的字符串是:%s\n", encrypted);
// 解密字符串
char decrypted[100];
strcpy(decrypted, encrypted);
for (size_t i = 0; decrypted[i]; ++i) {
decrypted[i] = decrypt(decrypted[i]);
}
// 输出解密后的字符串
printf("解密后的字符串是:%s\n", decrypted);
return 0;
}
```
这段代码首先包含了必要的头文件,然后定义了两个映射表。`encrypt()` 和 `decrypt()` 函数分别实现了加密和解密的功能。在`main()`函数中,用户被提示输入学号姓名,之后进行加密并输出,接着解密并将结果也输出。注意,在实际应用中,可能还需要处理一些边缘情况,比如输入超出长度限制等。
【相关问题】:
1. 如何处理用户输入的非字母字符?
2. 在加密过程中如果某个字符不存在于映射表中,应该如何处理?
3. 如何优化代码以提高性能?
阅读全文
相关推荐
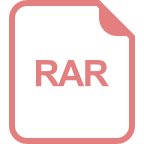
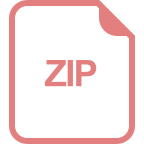
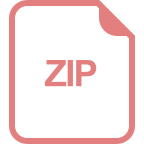
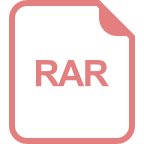
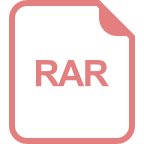
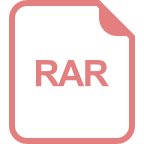
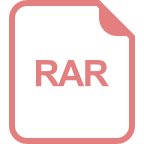
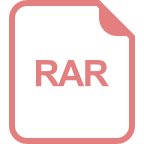
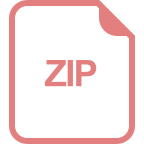
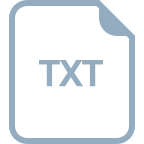
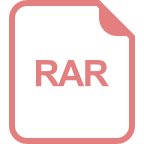
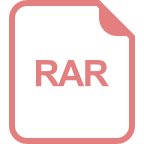
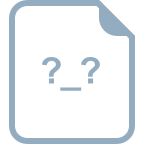
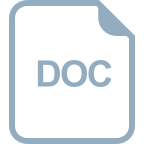
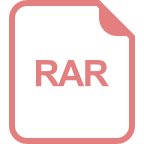
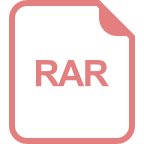
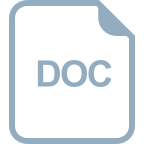
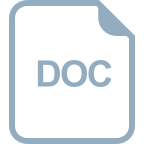
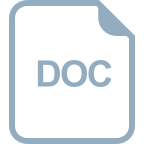