在C++中如何防止int转byte时的数据溢出?
时间: 2024-09-25 11:01:42 浏览: 29
在 C++ 中,为了避免将 `int` 类型转换为 `byte`(通常表示为 `char` 或 `signed char`)时发生数据溢出,你可以采取以下几种策略:
1. **范围检查**:在进行转换之前,检查 `int` 值是否在 `byte` 的有效范围内(通常是 -128 到 127 对于有符号 byte)。如果不在这个范围内,可以抛出异常或者处理为默认值。
```cpp
if (value >= std::numeric_limits<signed char>::min() && value <= std::numeric_limits<signed char>::max()) {
byte = static_cast<signed char>(value);
} else {
// 处理溢出,可能抛出异常或者设置默认值
}
```
2. **手动类型转换**:通过取模运算符 `%` 来确保数值落在 `byte` 的边界内,但这并不总是可靠的,因为如果输入值非常大,取模操作可能导致意外结果。
```cpp
byte = static_cast<signed char>(value % 256); // 这里假设 byte 是 unsigned char
```
3. **使用模板**:使用模板可以编写通用的函数,处理不同类型的转换,并在转换前进行适当的检查。
```cpp
template <typename TargetType>
TargetType safeToIntCast(int input) {
if (input > std::numeric_limits<TargetType>::max() || input < std::numeric_limits<TargetType>::min()) {
throw overflow_error("Input value out of range for target type");
}
return static_cast<TargetType>(input);
}
// 使用时:
byte = safeToIntCast<signed char>(your_int_value);
```
4. **类型别名和枚举**:如果 `int` 确实过大,可以定义一个新的类型别名,使其更精确地代表所需的范围,如 `std::uint8_t`。
```cpp
using Byte = std::uint8_t;
Byte byteValue = static_cast[Byte](your_int_value);
```
阅读全文
相关推荐
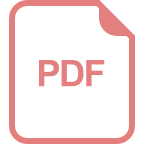
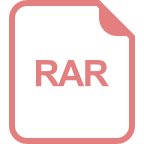
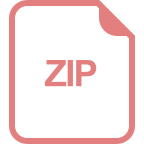
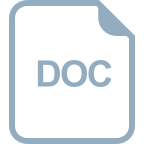
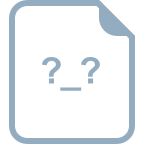
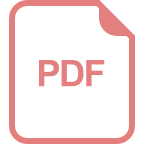
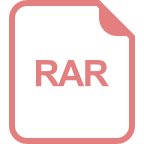
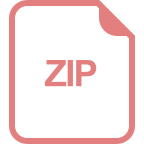
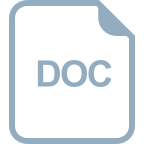
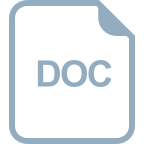
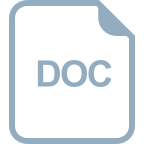
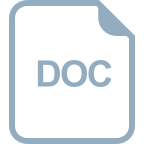
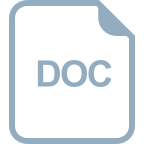
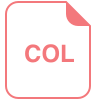
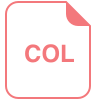
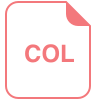


