asp.net添加Repository仓库模式 用vs2022版本写
时间: 2024-12-21 21:23:06 浏览: 3
ASP.NET中添加Repository模式主要是为了将数据访问层(DAL,Data Access Layer)从业务逻辑层(BL,Business Logic Layer)分离出来,提高代码的可维护性和复用性。Repository模式是一个软件设计模式,它定义了一个用于与数据源交互的接口,而实际的数据访问实现则由特定的实现类(如ADO.NET、Entity Framework等)负责。
以下是使用Visual Studio 2022创建Repository模式的基本步骤:
1. **创建接口**: 首先,在项目中创建一个名为`IRepository<T>`的通用接口,其中`T`是你需要操作的数据实体类型。例如,如果你有一个`Product`实体,可以这样定义:
```csharp
public interface IProductRepository
{
Task<List<Product>> GetAllProducts();
Task<Product> GetProductById(int id);
Task SaveProduct(Product product);
// 其他 CRUD 操作...
}
```
2. **数据访问类**: 创建一个实现了该接口的具体类,比如`ProductRepository`, 这里使用EF Core作为示例:
```csharp
public class ProductRepository : IProductRepository
{
private readonly DbContext _dbContext;
public ProductRepository(DbContext dbContext)
{
_dbContext = dbContext;
}
public async Task<List<Product>> GetAllProducts()
{
return await _dbContext.Products.ToListAsync();
}
// 使用DbContext的方法填充其他操作...
}
```
3. **注入到服务/控制器**: 在业务逻辑层中,通过依赖注入的方式获取`IProductRepository`实例,以便于在需要的时候调用它的方法:
```csharp
services.AddControllers().AddControllers(options => options.Filters.Add<AuthorizationFilter>());
services.AddScoped<IProductRepository, ProductRepository>();
```
4. **使用Repository**: 在控制器或服务类中,你可以像下面这样使用`IProductRepository`:
```csharp
private readonly IProductRepository _productRepository;
public ProductService(IProductRepository productRepository)
{
_productRepository = productRepository;
}
public async Task<ActionResult<IEnumerable<Product>>> GetProducts()
{
return await _productRepository.GetAllProducts();
}
```
阅读全文
相关推荐
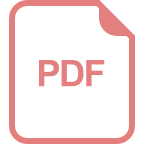
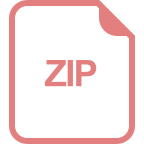
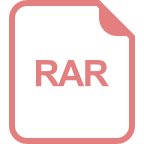
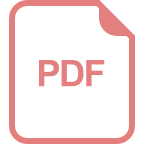
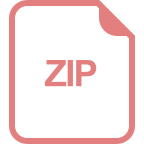
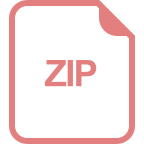
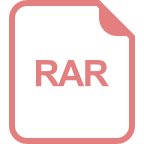
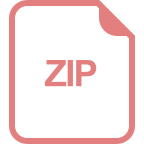
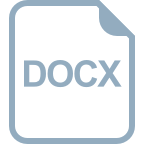
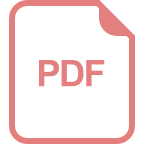
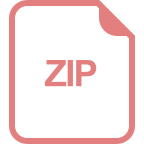
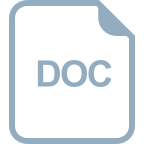
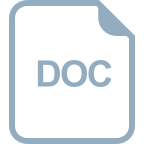
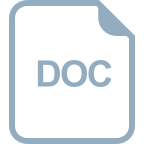
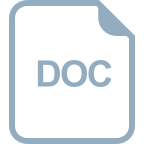
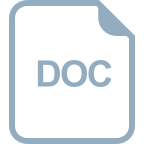
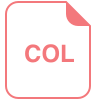
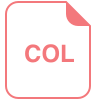