用vue中的watch()函数实现成绩表
时间: 2023-12-04 13:38:23 浏览: 41
在Vue中,可以使用watch()函数来监听数据的变化并执行相应的操作。如果要实现成绩表,可以先定义一个数组来存储学生的成绩信息,然后使用watch()函数来监听这个数组的变化,当数组中的数据发生变化时,可以重新计算总分和平均分并更新到页面上。
下面是一个简单的示例代码:
<template>
<div>
<table>
<thead>
<tr>
<th>姓名</th>
<th>语文</th>
<th>数学</th>
<th>英语</th>
<th>总分</th>
<th>平均分</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in scoreList" :key="index">
<td>{{ item.name }}</td>
<td><input type="number" v-model="item.chinese"></td>
<td><input type="number" v-model="item.math"></td>
<td><input type="number" v-model="item.english"></td>
<td>{{ item.total }}</td>
<td>{{ item.average }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import { reactive, watch } from 'vue'
export default {
setup() {
const scoreList = reactive([
{ name: '张三', chinese: 80, math: 90, english: 70, total: 0, average: 0 },
{ name: '李四', chinese: 70, math: 80, english: 90, total: 0, average: 0 },
{ name: '王五', chinese: 90, math: 70, english: 80, total: 0, average: 0 }
])
// 监听scoreList数组的变化
watch(scoreList, (newVal, oldVal) => {
newVal.forEach(item => {
item.total = item.chinese + item.math + item.english
item.average = (item.total / 3).toFixed(2)
})
}, { deep: true })
return {
scoreList
}
}
}
</script>
<style scoped>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: center;
}
th {
background-color: #f2f2f2;
}
</style>
相关推荐
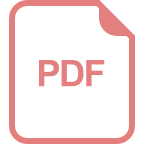
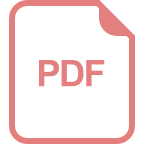
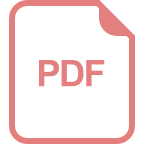
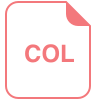
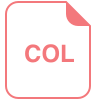
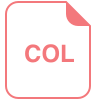
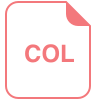
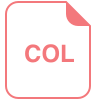









