rust编写出一个计算器
时间: 2024-09-10 08:12:06 浏览: 78
Rust是一种系统级编程语言,以其内存安全性和高性能而闻名。创建一个简单的命令行计算器在Rust中可以分为几个步骤:
首先,你需要设置一个项目并引入必要的`std`库,因为其中包含了基本的数学运算函数:
```rust
fn main() {
// Import necessary functions from std::io and std::num
use std::io;
use std::io::{self, BufRead};
use std::num::ops::Add;
let mut input = String::new();
io::stdin().read_line(&mut input).expect("Failed to read line");
}
```
接着,你可以设计一个计算函数,它接受两个字符串作为输入,解析成数字,并进行加法操作:
```rust
fn calculate(mut nums: String) -> i32 {
let mut first_num = String::new();
let mut second_num = String::new();
// Split the input by ' + ' or ' - '
for token in nums.split_whitespace() {
if token == "+" || token == "-" {
first_num.trim().parse::<i32>().expect("Invalid number");
second_num.trim().parse::<i32>().expect("Invalid number");
match token {
"+" => println!("{} + {} = {}", first_num, second_num, first_num.parse::<i32>() + second_num.parse::<i32>()),
_ => println!("{} - {} = {}", first_num, second_num, first_num.parse::<i32>() - second_num.parse::<i32>()),
_ => panic!("Unknown operator"),
}
first_num.clear();
second_num.clear();
} else {
second_num.push_str(token);
}
}
// If there's still a number at the end, parse it as the last operand
if !second_num.is_empty() {
first_num = second_num;
}
first_num.trim().parse::<i32>().expect("Invalid number") // Process the last remaining number
}
```
最后,在`main`函数中获取用户输入,调用`calculate`函数:
```rust
fn main() {
loop {
io::stdin()
.lock()
.lines()
.map(|line| line.unwrap())
.for_each(|input| {
let result = calculate(input);
println!("Result: {}", result);
});
print!("Enter another calculation (or type 'quit' to exit): ");
}
}
```
这个简单的计算器会持续接收用户的输入直到用户输入"quit"为止。
阅读全文
相关推荐
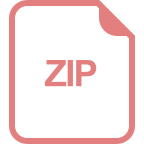
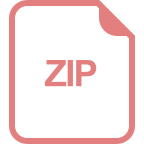
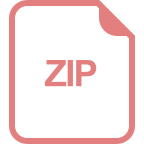
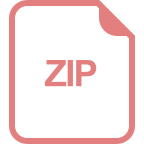


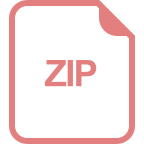
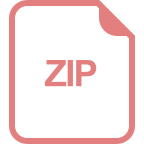
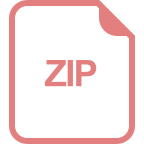
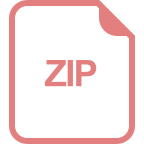
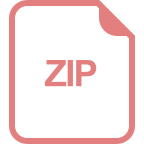
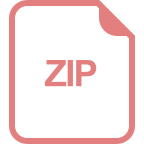
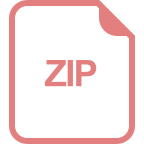
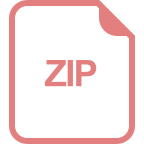
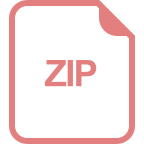
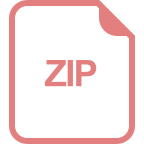
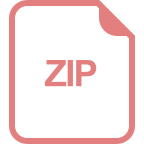


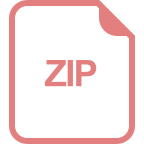