aes加密算法python代码,并详细解释,要求代码通俗易懂,适合python初学者
时间: 2024-10-25 22:15:16 浏览: 52
AES(Advanced Encryption Standard)是一种对称加密算法,用于保护数据的安全传输。Python中可以使用`cryptography`库来实现AES加密。以下是简单的AES加解密示例,包括初始化向量(IV)和密码(key),这些对于加密过程至关重要:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
# 导入密码
password = b"your_password_here"
salt = b"your_salt_here" # 可选,通常由应用生成并提供给用户
# 密码通过PBKDF2哈希以增强安全性
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32, # AES key size in bytes (128 bits)
salt=salt,
iterations=100000, # 越大越安全,但计算时间增加
backend=default_backend()
)
key = kdf.derive(password) # 密钥
# 对象初始化,选择AES-CTR模式因为它是流式的
cipher = Cipher(algorithms.AES(key), modes.CTR(backend=default_backend()), backend=default_backend())
# 加密函数
def encrypt(data):
encryptor = cipher.encryptor()
ct_bytes = encryptor.update(data) + encryptor.finalize()
return ct_bytes
# 解密函数
def decrypt(ciphertext):
decryptor = cipher.decryptor()
pt_bytes = decryptor.update(ciphertext) + decryptor.finalize()
return pt_bytes
# 示例:加密和解密字符串
plaintext = "Hello, World!"
ciphertext = encrypt(plaintext.encode('utf-8'))
decrypted_text = plaintext.decode() if ciphertext else None # 解密需要明文先编码
print(f"Ciphertext: {ciphertext.hex()}")
print(f"Decrypted Text: {decrypted_text}")
阅读全文
相关推荐



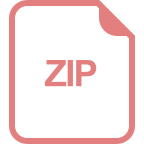


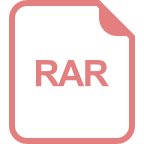





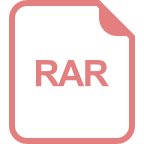