请用python帮我写一个代码,设计有 n 个进程共享 m 个系统资源的系统,基于银行家算法和安全性算法,进 程可动态的申请和释放资源,系统按各进程的申请动态的分配资源。要求打印资源动态分配矩阵
时间: 2024-11-03 14:20:33 浏览: 22
在Python中,我们可以使用`multiprocessing`库来模拟并发进程,并结合简单的内存模型来实现银行家算法。这里是一个简化版的示例,用于演示基本的概念,而不是完全实现了安全性和算法细节。这个例子并不包括实时的系统资源管理,而是假设了一个静态的资源池。
```python
import multiprocessing
# 假设我们有5个进程和3种类型的资源
processes = 5
resources = {
'type_1': {'max': 4, 'current': 0},
'type_2': {'max': 6, 'current': 0},
'type_3': {'max': 8, 'current': 0}
}
# 定义进程类,模拟申请、释放资源操作
class Process(multiprocessing.Process):
def __init__(self, id, reqs):
super().__init__()
self.id = id
self.requested = reqs
self.resources_granted = {k: 0 for k in reqs}
def run(self):
while True:
print(f"Process {self.id} requesting: {self.requested}")
granted = self._try_allocate_resources()
if not granted:
print(f"Process {self.id} cannot allocate resources, exiting.")
break
# 这里可以添加实际的任务或循环,这里仅做简单模拟
time.sleep(1)
self._release_resources()
def _try_allocate_resources(self):
max_resources = sum(resources[r]['max'] for r in self.requested)
available = all(r['current'] + self.requested[r] <= r['max'] for r in resources)
if available:
for resource, amount in self.requested.items():
resources[resource]['current'] += amount
self.resources_granted[resource] = amount
return True
else:
return False
def _release_resources(self):
for resource, amount in self.resources_granted.items():
resources[resource]['current'] -= amount
if resources[resource]['current'] < 0:
raise ValueError("Invalid release of resources")
# 创建进程并运行
requests = [[1, 2, 0], [2, 1, 0], [0, 0, 3], [2, 0, 1], [1, 1, 1]]
process_list = [Process(i+1, reqs) for i, reqs in enumerate(requests)]
for proc in process_list:
proc.start()
# 等待所有进程结束
for proc in process_list:
proc.join()
```
这个代码创建了几个进程,每个进程都有固定的资源需求。它们会尝试申请资源,在满足银行家算法的情况下进行分配。注意,这只是一个基础示例,真实环境中需要更复杂的锁机制来保证数据一致性,以及更严谨地处理资源状态更新。
阅读全文
相关推荐
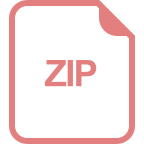
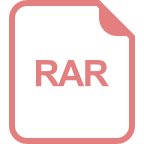
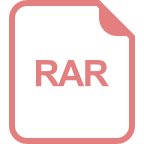

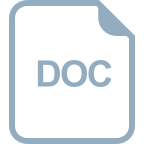
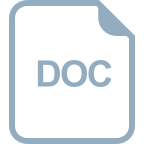
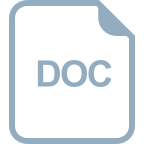
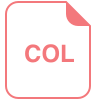
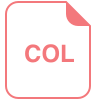
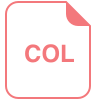
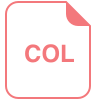
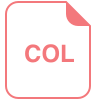
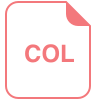
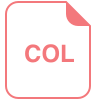
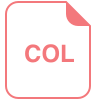
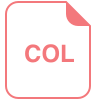
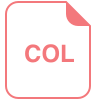
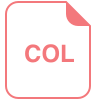
