unet图像分割pytorch代码手写数字识别
时间: 2024-10-05 21:01:01 浏览: 18
U-Net是一种经典的卷积神经网络架构,特别适用于像图像分割这样的任务,它广泛用于医学图像分析、遥感图像处理等领域。对于手写数字识别,虽然U-Net主要用于像素级别的分割,但它也可以作为特征提取部分与后续的全连接层结合,用于分类任务。
下面是一个简单的U-Net模型在PyTorch中用于手写数字识别的基本代码框架:
```python
import torch
from torch import nn
class UNet(nn.Module):
def __init__(self, in_channels=1, out_channels=10, num_classes=10):
super(UNet, self).__init__()
# 编码器部分
self.encoder = nn.Sequential(
nn.Conv2d(in_channels, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(2, 2),
nn.Conv2d(64, 128, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(2, 2),
nn.Conv2d(128, 256, kernel_size=3, padding=1),
nn.ReLU(),
nn.MaxPool2d(2, 2)
)
# 中间层
self.bottleneck = nn.Conv2d(256, 512, kernel_size=3, padding=1)
# 解码器部分
self.decoder = nn.Sequential(
nn.ConvTranspose2d(512, 256, kernel_size=2, stride=2),
nn.ReLU(),
nn.Conv2d(512, 256, kernel_size=3, padding=1),
nn.ReLU(),
nn.ConvTranspose2d(256, 128, kernel_size=2, stride=2),
nn.ReLU(),
nn.Conv2d(128, 64, kernel_size=3, padding=1),
nn.ReLU(),
nn.ConvTranspose2d(64, out_channels, kernel_size=2, stride=2) # 输出通道数等于类别数
)
self.classification_head = nn.Linear(128 * 4 * 4, num_classes) # 最后的分类层
def forward(self, x):
encodings = self.encoder(x)
bottleneck = self.bottleneck(encodings[-1])
decodings = self.decoder(bottleneck)
final = self.classification_head(decodings.reshape(-1, 128*4*4))
return final, decodings
# 使用模型实例
model = UNet()
```
注意,这只是一个基本版本,实际应用可能还需要添加数据预处理、损失函数、优化器以及训练和评估循环。此外,对于手写数字识别,通常会用到预训练的MNIST数据集,而不是直接用作图像分割任务。如果你想要做的是手写数字识别,可能需要调整网络结构并去掉最后的解码部分,专注于最后一层的分类任务。
相关推荐
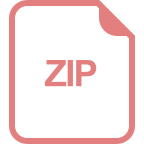
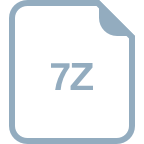
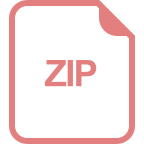

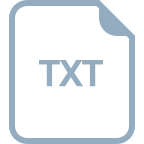
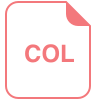
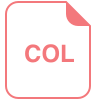
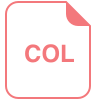
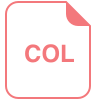
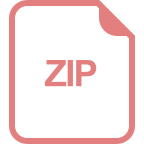
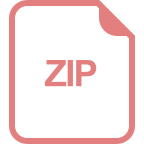
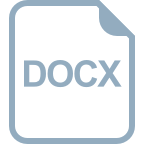
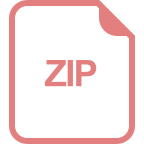
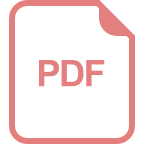
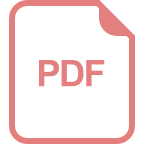