java ftpclient 连接池
时间: 2023-11-13 08:01:09 浏览: 151
Java FTPClient连接池是用于管理和维护FTP连接的资源池。它可以有效地管理FTP连接,减少因频繁创建和销毁连接所带来的性能损耗,提高系统的稳定性和可靠性。
连接池通常包括了连接的创建、初始化、销毁和回收等功能。它可以根据系统的实际需求动态地维护连接的数量,以便更好地利用系统资源。
Java FTPClient连接池的实现通常会采用线程安全的方式,以确保多个线程能够安全地使用连接池中的连接资源。同时,连接池也会对连接的空闲时间、超时时间等进行管理,以免因连接的长时间空闲造成资源的浪费。
使用Java FTPClient连接池可以有效地提高系统的性能和稳定性。它能够在高并发的情况下更好地管理FTP连接,避免了频繁创建和销毁连接所带来的开销,同时也能够更好地控制连接的数量,以防止过多的连接占用系统资源。
总之,Java FTPClient连接池是一个非常实用的工具,能够帮助开发人员更好地管理和维护FTP连接,提高系统的性能和稳定性。它是开发FTP应用程序时不可或缺的重要组件。
相关问题
springboot ftp连接池实现
以下是使用SpringBoot实现FTP连接池的步骤:
1.在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.7.0</version>
</dependency>
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2.创建FTP连接池配置类FtpClientPoolConfig,配置连接池的最大、最小连接数、连接超时时间等参数。
```java
@Configuration
@ConfigurationProperties(prefix = "ftp.pool")
@Data
public class FtpClientPoolConfig {
private int maxTotal;
private int maxIdle;
private int minIdle;
private long maxWaitMillis;
private boolean testOnBorrow;
private boolean testOnReturn;
private boolean testWhileIdle;
private long timeBetweenEvictionRunsMillis;
private int numTestsPerEvictionRun;
private long minEvictableIdleTimeMillis;
}
```
3.创建FTP连接池类FtpClientPool,使用Apache Commons Pool2实现连接池。
```java
@Component
public class FtpClientPool extends GenericObjectPool<FtpClient> {
public FtpClientPool(FtpClientFactory factory, FtpClientPoolConfig config) {
super(factory, new GenericObjectPoolConfig());
this.setMaxTotal(config.getMaxTotal());
this.setMaxIdle(config.getMaxIdle());
this.setMinIdle(config.getMinIdle());
this.setMaxWaitMillis(config.getMaxWaitMillis());
this.setTestOnBorrow(config.isTestOnBorrow());
this.setTestOnReturn(config.isTestOnReturn());
this.setTestWhileIdle(config.isTestWhileIdle());
this.setTimeBetweenEvictionRunsMillis(config.getTimeBetweenEvictionRunsMillis());
this.setNumTestsPerEvictionRun(config.getNumTestsPerEvictionRun());
this.setMinEvictableIdleTimeMillis(config.getMinEvictableIdleTimeMillis());
}
}
```
4.创建FTP连接池工厂类FtpClientFactory,用于创建FTP连接对象。
```java
@Component
public class FtpClientFactory extends BasePooledObjectFactory<FtpClient> {
private FtpClientProperties properties;
public FtpClientFactory(FtpClientProperties properties) {
this.properties = properties;
}
@Override
public FtpClient create() throws Exception {
FtpClient ftpClient = new FtpClient();
ftpClient.connect(properties.getHost(), properties.getPort());
ftpClient.login(properties.getUsername(), properties.getPassword());
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
ftpClient.setBufferSize(properties.getBufferSize());
ftpClient.setControlEncoding(properties.getEncoding());
ftpClient.enterLocalPassiveMode();
return ftpClient;
}
@Override
public PooledObject<FtpClient> wrap(FtpClient ftpClient) {
return new DefaultPooledObject<>(ftpClient);
}
@Override
public void destroyObject(PooledObject<FtpClient> p) throws Exception {
FtpClient ftpClient = p.getObject();
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
}
@Override
public boolean validateObject(PooledObject<FtpClient> p) {
FtpClient ftpClient = p.getObject();
try {
return ftpClient.sendNoOp();
} catch (IOException e) {
return false;
}
}
}
```
5.创建FTP连接池属性类FtpClientProperties,用于配置FTP连接的相关参数。
```java
@ConfigurationProperties(prefix = "ftp")
@Data
public class FtpClientProperties {
private String host;
private int port;
private String username;
private String password;
private int bufferSize;
private String encoding;
}
```
6.在application.yml文件中配置FTP连接池的相关参数。
```yaml
ftp:
host: ftp.example.com
port: 21
username: username
password: password
bufferSize: 1024
encoding: UTF-8
ftp:
pool:
maxTotal: 10
maxIdle: 5
minIdle: 1
maxWaitMillis: 3000
testOnBorrow: true
testOnReturn: false
testWhileIdle: true
timeBetweenEvictionRunsMillis: 60000
numTestsPerEvictionRun: -1
minEvictableIdleTimeMillis: 1800000
```
7.在需要使用FTP连接的地方,注入FtpClientPool对象,从连接池中获取FTP连接对象。
```java
@Service
public class FtpService {
@Autowired
private FtpClientPool ftpClientPool;
public void uploadFile(String remotePath, String fileName, InputStream inputStream) throws Exception {
FtpClient ftpClient = ftpClientPool.borrowObject();
try {
ftpClient.changeWorkingDirectory(remotePath);
ftpClient.storeFile(fileName, inputStream);
} finally {
ftpClientPool.returnObject(ftpClient);
}
}
}
```
springboot中ftp连接池
Spring Boot中可以通过使用Apache Commons Net库来实现FTP连接池。下面是实现FTP连接池的步骤和代码示例:
1. 首先,需要在pom.xml文件中添加Apache Commons Net依赖:
```xml
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2. 创建一个FTP连接池管理类,用于管理和维护FTP连接池。可以使用Apache Commons Pool库来实现连接池的功能:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.pool2.ObjectPool;
import org.apache.commons.pool2.impl.GenericObjectPool;
public class FTPPoolManager {
private static ObjectPool<FTPClient> pool;
static {
// 创建FTPClientFactory实例,用于创建FTPClient对象
FTPClientFactory factory = new FTPClientFactory();
// 配置连接池
GenericObjectPool.Config config = new GenericObjectPool.Config();
config.setMaxTotal(10); // 设置连接池最大连接数
config.setMaxIdle(5); // 设置连接池最大空闲连接数
config.setMinIdle(1); // 设置连接池最小空闲连接数
// 创建连接池
pool = new GenericObjectPool<>(factory, config);
}
public static FTPClient borrowObject() throws Exception {
return pool.borrowObject();
}
public static void returnObject(FTPClient ftpClient) {
pool.returnObject(ftpClient);
}
}
```
3. 创建一个FTPClient工厂类,用于创建FTPClient对象:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.pool2.BasePooledObjectFactory;
public class FTPClientFactory extends BasePooledObjectFactory<FTPClient> {
@Override
public FTPClient create() throws Exception {
// 创建FTPClient对象
FTPClient ftpClient = new FTPClient();
ftpClient.connect("ftp.example.com", 21); // 连接FTP服务器
ftpClient.login("username", "password"); // 登录FTP服务器
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE); // 设置传输模式为二进制
return ftpClient;
}
@Override
public void destroyObject(FTPClient ftpClient) throws Exception {
ftpClient.logout(); // 退出登录
ftpClient.disconnect(); // 断开连接
}
@Override
public boolean validateObject(FTPClient ftpClient) {
boolean result = false;
try {
result = ftpClient.sendNoOp(); // 发送NOOP命令检测连接是否有效
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
```
4. 在使用FTP连接的地方,可以通过FTP连接池管理类来获取FTPClient对象,并使用完后归还给连接池:
```java
import org.apache.commons.net.ftp.FTPClient;
public class FTPExample {
public static void main(String[] args) {
FTPClient ftpClient = null;
try {
ftpClient = FTPPoolManager.borrowObject(); // 从连接池中获取FTPClient对象
// 在这里执行FTP操作,如上传文件、下载文件等
} catch (Exception e) {
e.printStackTrace();
} finally {
if (ftpClient != null) {
FTPPoolManager.returnObject(ftpClient); // 将FTPClient对象归还给连接池
}
}
}
}
```
通过以上步骤和代码示例,我们可以在Spring Boot中实现FTP连接池,以提高FTP连接的性能和效率。
阅读全文
相关推荐
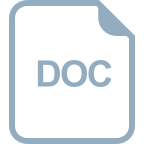
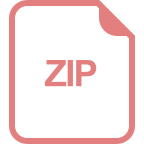
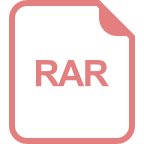
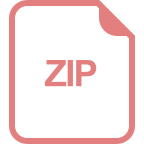
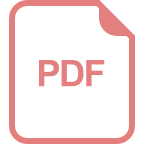
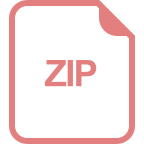
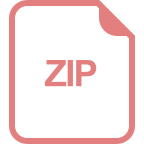
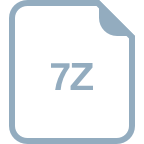
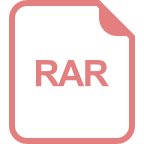
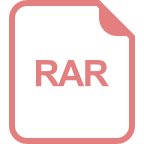
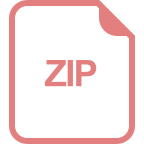
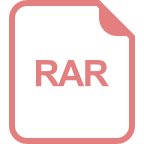
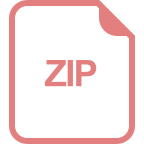
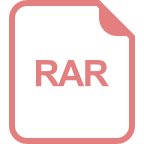