如何在WPF中使用MVVM架构设计模式来选取一个文件夹,并加载该文件夹内的所有BMP图像文件?
时间: 2024-11-06 13:17:45 浏览: 14
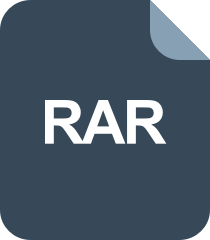
在WPF的MVVM架构中,用OxyPlot绘制曲线过程
在Windows Presentation Foundation (WPF) 中使用 Model-View-ViewModel (MVVM) 架构设计模式来选取文件夹并加载其中的BMP图像文件,你需要按照以下步骤操作:
1. **模型(Model)**: 创建一个 `ImageFolderModel` 或类似的类,它包含一个属性用于存储选定的文件夹路径。当用户选择文件夹时,这个模型应该能够获取并更新路径。
```csharp
public class ImageFolderModel
{
public string SelectedFolderPath { get; set; }
// 可能需要添加一个方法来列出文件夹中的BMP文件
public List<string> GetBmpFiles()
{
return Directory.GetFiles(SelectedFolderPath, "*.bmp").ToList();
}
}
```
2. **视图(View)**: 使用WPF的 `System.Windows.Forms.OpenFileDialog` 控件让用户选择文件夹。选中文件夹后,将结果传递给 VM。
```xaml
<Button Content="选择文件夹" Command="{Binding SelectFolderCommand}" />
<TextBox Text="{Binding SelectedFolderPath, UpdateSourceTrigger=PropertyChanged}"/>
<ListBox ItemsSource="{Binding BmpFiles}">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding}" />
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
```
3. **视图模型(ViewModel)**: 创建 `ImageFolderViewModel` 类,这里包含方法处理文件夹选择命令,以及订阅到模型的变化。
```csharp
using GalaSoft.MvvmLight.Command;
public class ImageFolderViewModel : ViewModelBase
{
private readonly ImageFolderModel _model;
public ImageFolderViewModel(ImageFolderModel model)
{
_model = model;
SelectFolderCommand = new RelayCommand(() => _model.SelectFolder());
}
public RelayCommand<SelectFolderCommandParameters> SelectFolderCommand { get; }
public string SelectedFolderPath => _model.SelectedFolderPath;
// 如果你想通过命令获取BMP文件列表
public List<string> BmpFiles => _model.GetBmpFiles();
}
```
4. **数据绑定(Data Binding)**: 将 VM 和 UI 绑定起来,比如通过 GalaSoft.MvvmLight 框架。
5. **事件通知(Event Notifications)**: MVVM 通常会通过观察者模式来通知 UI 更新,例如当模型改变时,会触发 UI 的更新。
阅读全文
相关推荐
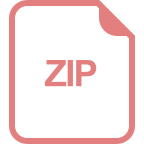
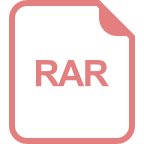
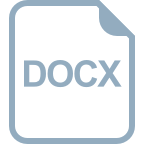
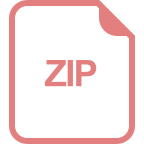
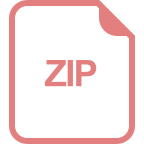
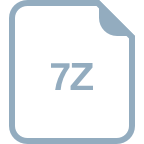
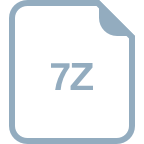
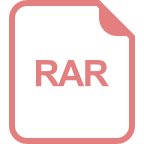
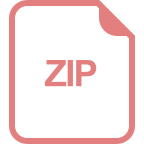
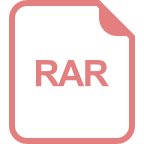
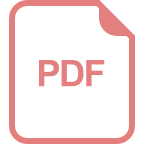
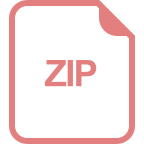
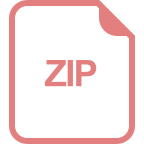
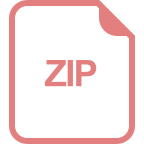
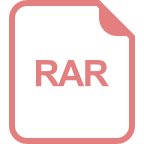
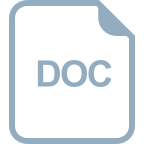