为学生管理系统设置一个密码C语言
时间: 2024-10-28 15:12:04 浏览: 7
在C语言中,为学生管理系统设置密码通常涉及到用户输入验证和存储安全性的考虑。下面是一个简单的示例,展示了如何让用户创建并确认密码:
```c
#include <stdio.h>
#include <string.h> // 使用字符串库
#define PASSWORD_LENGTH 8 // 密码最小长度为8字符
// 函数声明
void create_password(char *password);
void confirm_password(char *new_password, char *confirm);
int main() {
char student_password[PASSWORD_LENGTH + 1]; // 存储密码的数组
char confirm_password[PASSWORD_LENGTH + 1];
printf("请输入您的新密码: ");
fgets(student_password, PASSWORD_LENGTH + 1, stdin); // 防止换行符
student_password[strlen(student_password) - 1] = '\0'; // 去掉多余的换行符
printf("请再次输入密码进行确认: ");
fgets(confirm_password, PASSWORD_LENGTH + 1, stdin);
confirm_password[strlen(confirm_password) - 1] = '\0';
// 验证密码是否一致
if (strcmp(student_password, confirm_password) == 0) {
create_password(student_password);
printf("密码已设置成功!\n");
} else {
printf("两次输入的密码不一致,请重试。\n");
}
return 0;
}
void create_password(char *password) {
// 这里可以对密码做加密处理,这里仅作简单保存
FILE *file = fopen("student_password.txt", "w"); // 打开文件,假设存在学生信息文件
fprintf(file, "%s", password);
fclose(file);
printf("密码已保存到文件中.\n");
}
void confirm_password(char *new_password, char *confirm) {
// 这里用于提示密码已确认,实际应用会更复杂
printf("您确认的密码是:%s\n", new_password);
}
```
阅读全文
相关推荐





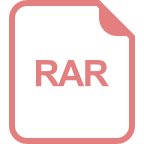


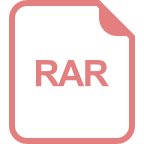
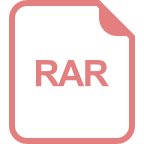







