输入有序表中元素的个数n,占一行;按从小到大的顺序输入n个整数,以空格隔开,占一行;输入待插入元素x,y占一行。输出插入x,y之后的有序表,用空格隔开,最后一个整数后面要求有空格,请用c语言实现这个程序
时间: 2024-09-08 08:02:24 浏览: 71
以下是一个用C语言实现的程序,该程序根据用户输入的有序数组大小和元素,然后接收两个待插入元素,并将这两个元素插入到数组中,最后输出新的有序数组。
```c
#include <stdio.h>
// 函数声明
void insertElements(int arr[], int n, int x, int y, int *newSize);
int main() {
int n, x, y;
// 读取有序数组的大小
scanf("%d", &n);
int arr[n + 2]; // 数组长度为n+2,为插入两个元素预留空间
// 读取有序数组的元素
for (int i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
// 读取待插入的两个元素
scanf("%d %d", &x, &y);
// 调用函数进行插入操作,并更新数组的大小
int newSize;
insertElements(arr, n, x, y, &newSize);
// 输出新的有序数组
for (int i = 0; i < newSize; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
// 函数定义
void insertElements(int arr[], int n, int x, int y, int *newSize) {
// 找到插入位置
int pos = n;
while (pos > 0 && arr[pos - 1] > x) {
arr[pos] = arr[pos - 1]; // 向后移动元素
pos--;
}
arr[pos] = x; // 插入x
// 找到y的插入位置,注意y的值可能已经插入过了,因此从n+1开始
pos = n + 1;
while (pos > 0 && arr[pos - 1] > y) {
arr[pos] = arr[pos - 1]; // 向后移动元素
pos--;
}
arr[pos] = y; // 插入y
*newSize = n + 2; // 更新数组大小
}
```
请注意,这个程序假设输入的数组已经是有序的,并且输入的两个待插入元素x和y也是按照升序给出的。如果待插入元素已经存在于数组中,那么程序将它们视为不同的元素进行插入操作。如果数组中不允许重复元素,你需要添加额外的逻辑来检查并避免重复。
阅读全文
相关推荐
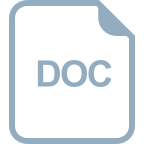
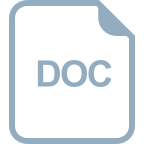
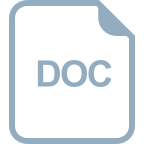











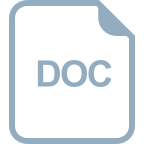
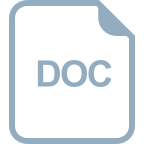
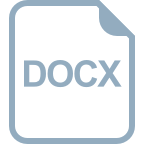