springboot装饰器模式
时间: 2023-10-12 07:58:33 浏览: 242
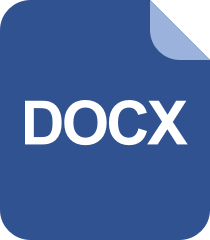
装饰器模式
在Spring Boot中使用装饰器模式,可以通过自定义注解和切面来实现。下面是一个简单的示例:
首先,创建一个自定义注解`@Log`,用于标记需要记录日志的方法:
```java
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Log {
}
```
然后,创建一个切面类`LogAspect`,在该类中编写装饰器逻辑:
```java
@Aspect
@Component
public class LogAspect {
private static final Logger logger = LoggerFactory.getLogger(LogAspect.class);
@Around("@annotation(com.example.demo.Log)")
public Object logAround(ProceedingJoinPoint joinPoint) throws Throwable {
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
String methodName = method.getName();
logger.info("Before method execution: " + methodName);
Object result = joinPoint.proceed();
logger.info("After method execution: " + methodName);
return result;
}
}
```
在上面的代码中,我们使用了`@Around`注解来定义环绕通知,它会在被`@Log`注解标记的方法执行前后执行。在方法执行前,我们记录了方法名,并在方法执行后再次记录。
最后,我们可以在需要应用装饰器模式的方法上加上`@Log`注解:
```java
@RestController
public class MyController {
@Log
@GetMapping("/hello")
public String hello() {
return "Hello, world!";
}
}
```
这样,在访问`/hello`接口时,日志将会被记录。
这只是一个简单的示例,实际应用中可能会有更复杂的装饰器逻辑。你可以根据具体需求来定义自己的装饰器模式。希望对你有所帮助!
阅读全文
相关推荐
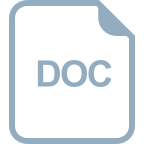
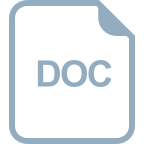
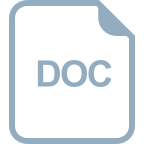


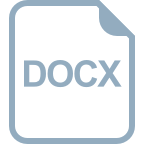
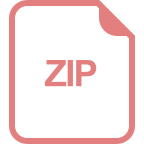
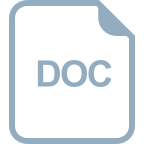
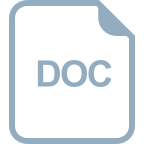
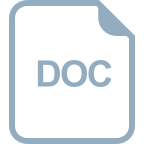
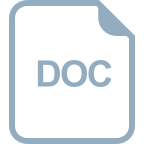
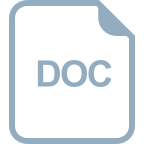
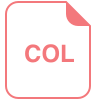


