sklearn preprocessing中,哪些情况选择Normalizer而非StandardScaler
时间: 2024-12-24 21:38:37 浏览: 4
在`sklearn.preprocessing`中,`StandardScaler`通常用于将数值特征缩放至均值为0,方差为1的标准正态分布。然而,在某些特定情况下,可能选择`Normalizer`而非`StandardScaler`:
1. **当不需要对整个特征集施加零均值和单位方差时**:如果你的数据集中心趋势不明显(非零均值),或者对数据的尺度变化不太敏感,`Normalizer`可以按列(l2范数)或按行(l1范数)进行规范化,使得每个特征向量的长度保持一致。
2. **处理稀疏数据**:对于稀疏矩阵,如基于文档的TF-IDF表示,`Normalizer`在保留稀疏性的同时进行归一化,而`StandardScaler`可能会转化为密集数组。
3. **保留原始数据分布的形状**:如果数据本身就有某种自然的比例关系,比如概率密度函数,`Normalizer`能更好地保持这种结构。
```python
from sklearn.preprocessing import Normalizer
# 假设我们有一个稀疏数据矩阵sparse_data
normalizer = Normalizer(norm='l2') # 使用l2范数
normalized_data = normalizer.transform(sparse_data)
```
相关问题
请举例讲解sklearn preprocessing中normalizer函数的应用,以及与standardscaler的区别
`sklearn.preprocessing`库中的`Normalizer`和`StandardScaler`都是预处理工具,但它们的作用不同。
`Normalizer`主要用于规范化数据,使得每个样本向量的欧几里得长度保持一致。它主要有两个参数:
- `norm`: 可选参数,如'l2'代表L2范数(默认),使每个特征向量除以其L2范数(即向量的平方和的平方根),这样每个特征具有单位欧几里得长度。这对于那些特征之间尺度差异大的情况非常有用,比如文档向量化[^1]。
- `copy`: 是否创建原数据的副本,默认为True,以防修改原数据。
例如,如果你有一个文本数据集,每个文档可以看作是一个词频向量,通过`Normalizer(norm='l2')`可以标准化这些向量,使得每个文档的重要性由其在整个语料库中的相对重要性来衡量,而不是由单词的数量决定。
相比之下,`StandardScaler`(也称为Z-score标准化)则是将数据缩放到均值为0,标准差为1的标准正态分布。这适用于数值特征,它会调整整个特征列,而不是单个样本的长度。
举个例子:
```python
from sklearn.preprocessing import Normalizer
# 假设data是一个包含词频矩阵的数组
normalizer = Normalizer()
normalized_data = normalizer.transform(data)
# 对于StandardScaler
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
standardized_data = scaler.fit_transform(data)
```
sklearn.preprocessing
The sklearn.preprocessing module provides various functions for preprocessing data before it can be used for machine learning. Some of the functions provided by this module are:
1. StandardScaler: This function scales the data such that it has zero mean and unit variance.
2. MinMaxScaler: This function scales the data to a specified range, usually between 0 and 1.
3. RobustScaler: This function scales the data by removing the median and scaling it to the interquartile range.
4. Normalizer: This function scales each sample to have a unit norm.
5. Binarizer: This function converts numerical features into binary features based on a threshold value.
6. LabelEncoder: This function converts categorical variables into numerical variables by assigning a unique label to each category.
7. OneHotEncoder: This function converts categorical variables into binary variables by creating new columns for each category.
8. PolynomialFeatures: This function creates new features by combining existing features using polynomial functions.
These functions can be used to preprocess data for various machine learning models such as linear regression, logistic regression, support vector machines, etc.
阅读全文
相关推荐
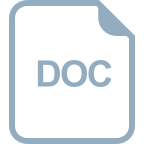
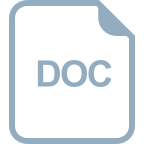
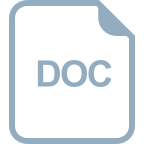
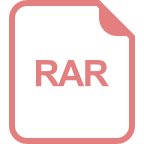
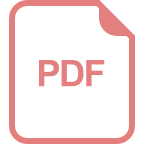
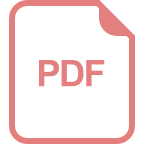
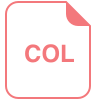
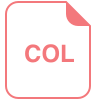
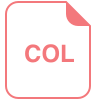
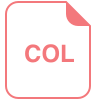
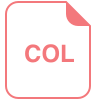
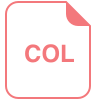
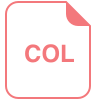
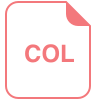


