【问题描述】输入日期的年份和月份,求该月有多少天。提示:对于月份为1、3、5、7、8、10、12的月份天数为31,月份为4、6、9、11的月份天数为30,月份为2时要结合年份考虑闰年的情况,编写程序
时间: 2024-10-29 08:24:07 浏览: 52
这是一个简单的编程任务,通常在处理日期计算或者验证日期有效性时遇到。在Python中,你可以使用`calendar`模块来解决这个问题。以下是用Python编写的示例函数:
```python
import calendar
def days_in_month(year, month):
# 判断是否为闰年
if month == 2:
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
return 29
else:
return 28
elif month in [1, 3, 5, 7, 8, 10, 12]:
return 31
else:
return 30
# 使用示例
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
days = days_in_month(year, month)
print(f"{year}年的{month}月有{days}天。")
```
在这个函数中,我们首先检查如果月份是2,就根据闰年的规则判断2月的天数;然后分别给其他非二月的月份分配固定的天数。
相关问题
用python写代码:题目描述:输入日期的年份和月份,求该月有多少天。提示:对于月份为1、3、5、7、8、10、12的月份天数为31,月份为4、6、9、11的月份天数为30,月份为2时要结合年份考虑闰年的情况。
以下是求该月份天数的 Python 代码:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
if month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
elif month == 2:
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
days = 29
else:
days = 28
else:
print("输入的月份不合法!")
if days:
print("{}年{}月有{}天。".format(year, month, days))
```
其中,`input()` 函数用于接收用户输入的年份和月份,`int()` 函数将输入的字符串转换为整数。`if...elif...else...` 结构用于判断该月份的天数,根据不同的情况赋值给 `days` 变量。对于二月份,需要额外考虑闰年的情况,通过判断年份是否是 4 的倍数、但不是 100 的倍数,或者是 400 的倍数,来确定该年是否是闰年。最后,如果 `days` 变量有值,就输出该月份的天数。
C语言请用switch语句进行程序设计,从键盘上输入一个指定年份和月份的日期,日期格式为:2018-7,判断出该月份有几天。 注:本题涉及到闰年、平年的判断,闰年的2月有29天,平年的2月有28天,已知符合下列条件之一者是润年: (1)能被4整除,但不能被100整除; (2)能被400整除。 输入输出描述如下: 输入特定日期的提示信息:"Please Input the Date:" 输入按题目要求格式的日期:"%d-%d" 输出该月份的天数n:"%d days" 如果输入数据格式有误:"Input error!\n"
好的,以下是您需要的C语言代码:
```c
#include <stdio.h>
int main()
{
int year, month, days;
printf("Please Input the Date:");
if (scanf("%d-%d", &year, &month) != 2) // 判断输入格式是否正确
{
printf("Input error!\n");
return 0;
}
switch (month)
{
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days = 31;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) // 判断是否为闰年
{
days = 29;
}
else
{
days = 28;
}
break;
default:
printf("Input error!\n");
return 0;
}
printf("%d days", days);
return 0;
}
```
希望能够帮到您!
阅读全文
相关推荐
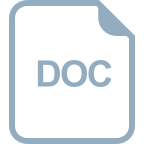
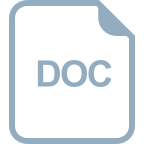
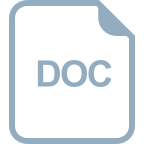
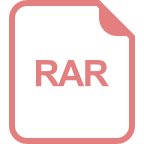
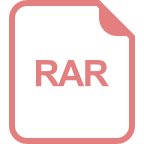
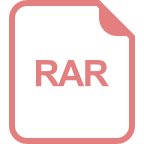










