vue 跳转页面跳出一级路由
时间: 2024-07-03 10:00:53 浏览: 162
在Vue中,如果你想从一个组件内部跳转到另一个页面并且不保留当前的路由,也就是不添加到当前的一级路由中,你可以使用`this.$router.push()`方法,并且传入一个不含父级路径的对象。例如,如果你有一个`/parentComponent`的父路由,你可以这样跳转:
```javascript
// 假设你要去的页面路径是 '/childComponent'
this.$router.push({ path: '/childComponent', name: 'childPage' }); // 如果有name属性,也可以用name代替path
```
这样只会导航到`childComponent`,不会增加到当前的`/parentComponent`路径下。如果`childComponent`是一个独立的路由,那么它会替换当前的路由,如果它是在其他路由的嵌套中,它会在那个路由内展开。
相关问题
vue3+ts在elementplusadmin框架路由跳转<keep-alive>不刷新视频流如何保留内容不刷新
在Vue3 + TypeScript环境中,如果你正在使用Element Plus Admin框架并遇到了`<keep-alive>`组件下路由切换时视频流未刷新的问题,这通常是为了在用户短时间内返回先前页面时保持页面状态,包括视频播放状态。要实现这一点,你需要做以下几步:
1. **设置组件守卫** (Route Guards):
在Vue Router的配置文件里,你可以使用`beforeEnter`或`meta`属性来判断是否需要保存当前视频的状态。例如:
```typescript
import { useRouter } from 'vue-router';
// 定义一个守卫函数
const videoGuard = async (to, from, next) => {
const router = useRouter();
if (router.meta.isKeepAlive && videoComponentRef.value?.ready) {
await videoComponentRef.value.pause(); // 停止视频播放
}
next();
};
// 使用该守卫
routes.forEach(route => {
route.meta.isKeepAlive = true; // 标记需要被 keep-alive 的路由
if (route.name === 'VideoRoute') {
route.beforeEnter = videoGuard;
}
});
```
2. **处理组件状态**:
在视频组件中,维护一个引用和状态管理(如Vuex)。当组件挂载到 `<keep-alive>` 中时,检查是否应该恢复视频播放:
```typescript
import { ref, onMounted } from 'vue';
import store from '@/store';
const videoComponentRef = ref(null);
const shouldPlayOnEnter = ref(false);
onMounted(() => {
// 当组件进入 keep-alive 区域,从 store 获取是否应立即播放
if (store.state.videoShouldPlayOnEnter) {
shouldPlayOnEnter.value = true;
}
});
beforeUnmount(() => {
// 跳出 keep-alive 时暂停视频
if (videoComponentRef.value) {
videoComponentRef.value.pause();
}
});
// 播放控制方法
const playVideo = () => {
if (shouldPlayOnEnter.value && videoComponentRef.value?.ready) {
videoComponentRef.value.play();
}
};
```
3. **在`<keep-alive>`标签中使用`v-if`动态显示组件**:
如果你想要更精确地控制何时将视频组件放入缓存,可以在`<keep-alive>`内使用`v-if`,比如:
```html
<keep-alive>
<component :is="currentComponent" v-if="$route.meta.isKeepAlive"></component>
</keep-alive>
```
**相关问题--:**
1. Vue Router的`beforeEnter`钩子是如何工作的?
2. 如何在Element Plus中使用Vuex管理组件状态?
3. 是否有其他方式避免视频在`<keep-alive>`外加载时初始化?
vue里面如何写一个跳出框架之外页面
在Vue.js中,如果你想从当前组件跳转到浏览器的根URL或者外部链接,可以使用`window.location`对象。以下是一个简单的例子:
```javascript
// 在模板中通常通过点击事件触发
<button @click="goToExternalPage">跳转到外部页面</button>
// 在methods部分定义方法
methods: {
goToExternalPage() {
// 跳转到一个新的URL
window.location.href = "https://www.example.com";
// 或者如果你只是想离开当前应用(SPA单页应用),可以用
// window.location.replace("https://www.example.com");
// 这会替换当前的历史记录,而不是添加新的条目
}
}
```
在这个例子中,当你点击按钮时,就会打开新标签页跳转到指定的URL。请注意,由于安全原因,对于一些敏感操作,如支付、登录等,最好避免直接使用`location`进行跳转,而是使用路由系统或者Vuex等官方推荐的方式管理状态。
阅读全文
相关推荐
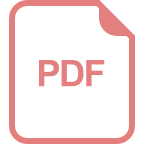
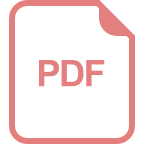
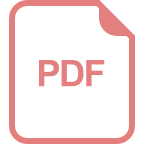
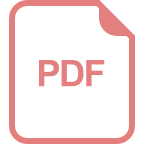
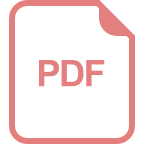
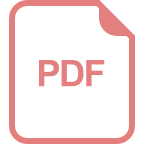
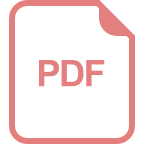
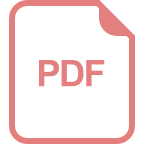
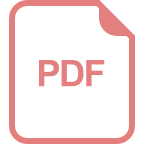







