利用几个例子教我学习c++
时间: 2024-11-27 15:09:32 浏览: 4
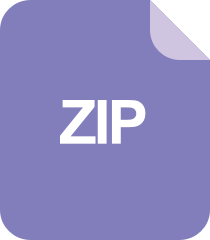
C、C++和Wolfram交互的初级教程和例子.zip

在C++中,学习基本语法和概念可以通过一些简单例子开始。这里有几个基础示例:
1. **变量声明和赋值**:
```cpp
int age; // 声明一个整型变量age
age = 25; // 赋值给age
cout << "Your age is: " << age << endl; // 输出变量的值
```
2. **条件语句 (if-else)**:
```cpp
bool isStudent = true;
if (isStudent) {
cout << "You are a student." << endl;
} else {
cout << "You are not a student." << endl;
}
```
3. **循环 (for loop)**:
```cpp
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i + 1 << endl;
}
```
4. **函数 (Function)**:
```cpp
void sayHello() {
cout << "Hello, World!" << endl;
}
sayHello(); // 调用函数
```
5. **数组 (Array)**:
```cpp
int numbers[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
cout << "Element at index " << i << " is: " << numbers[i] << endl;
}
```
6. **指针 (Pointer)**:
```cpp
int* ptr = new int(10);
cout << "Value pointed by pointer: " << *ptr << endl;
delete ptr; // 释放内存
```
7. **对象和成员函数 (Object and Methods)**:
```cpp
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
Dog dog;
dog.bark();
```
通过以上实例,你可以逐渐理解和应用C++的基本构造。记住,实践是学习的关键,尝试编写自己的程序并解决实际问题会有很大帮助。
阅读全文
相关推荐
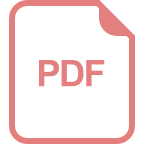
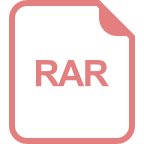
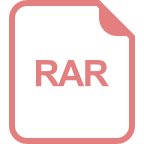
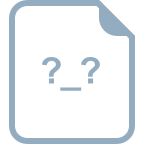
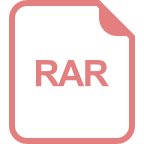
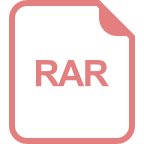
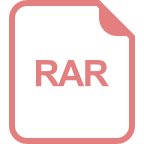
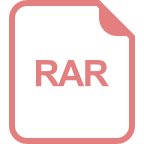
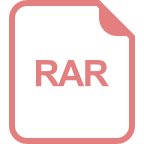
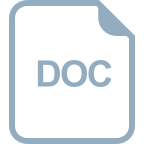
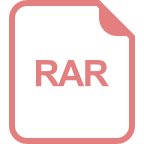
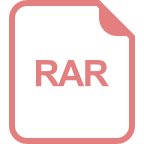
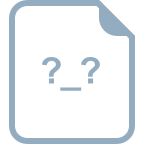
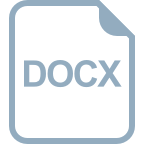
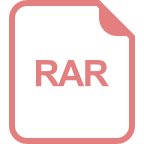
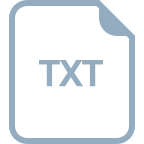
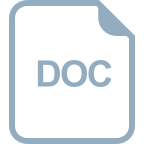
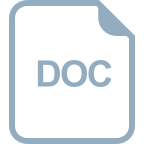