基于蚁群算法的组合优化问题求解
时间: 2024-12-31 20:42:33 浏览: 3
### 蚁群算法解决组合优化问题的方法
#### 4.2 ACO算法的基本思想
蚁群算法是一种基于群体智能的启发式搜索方法,旨在模仿真实世界中蚂蚁觅食行为中的自组织特性。当面对复杂的环境时,单只蚂蚁可能无法高效地找到食物源到巢穴的最佳路径;然而,在集体协作下,整个蚁群能够快速适应并发现较优解决方案。
具体来说,每一只虚拟蚂蚁根据当前节点连接至其他未访问过的城市之间的距离以及这些边上的信息素浓度决定下一步前往哪个城市。随着迭代次数增加,更短路径上累积的信息素量会相对更多,从而吸引更多后来者沿着相同路线前进,形成正反馈机制促进全局最优解收敛过程[^1]。
#### 使用蚁群算法求解旅行商问题(TSP)
对于经典的TSP而言,目标是在给定一组城市的前提条件下找出经过每一个地点恰好一次且回到起点的一条最短闭合回路。应用ACO框架处理该类难题涉及以下几个方面:
- **初始化参数设置**:定义初始时刻各路段所含有的信息素水平τij(0),通常设为常数C;设定期望启发因子α、信息素重要程度β以及其他控制探索与开发平衡度的相关系数。
- **构建解的过程**:按照概率公式\[ p_{ij}^{k}(t)=\frac{\left[\tau_{ij}(t)\right]^{\alpha}\left[\eta_{ij}\right]^{\beta}}{\sum_{l \in J_k(t)}\left[\tau_{il}(t)\right]^{\alpha}\left[\eta_{il}\right]^{\beta}} \](其中η代表启发函数值即两点间倒数距离)挑选下一个访问的城市直到完成一轮遍历全部顶点的操作为止。
- **更新规则**:依据新产生的可行方案质量调整对应弧段上的残留痕迹强度Δτij=Q/Lbest(L表示总路程长度,Q是预置比例因子), 同步考虑蒸发效应使得旧有记录随时间减弱。\(\tau_{ij}(t+1)=(1-\rho) * \tau_{ij}(t)+\Delta \tau_{ij}\)(ρ∈(0,1)指代衰减率)
```python
import numpy as np
from random import randint
class AntColonyOptimization:
def __init__(self, cities_distances, num_ants, alpha=1, beta=5, rho=.1, q=100):
self.cities_distances = cities_distances
self.num_cities = len(cities_distances)
self.pheromone_matrix = [[1 / (num_ants * self.num_cities)] *
self.num_cities for _ in range(self.num_cities)]
self.alpha = alpha
self.beta = beta
self.rho = rho
self.q = q
self.best_tour_length = float('inf')
self.best_tour = []
def select_next_city(self, current_city, unvisited_cities):
probabilities = []
total_prob = 0
for city in unvisited_cities:
pheromone_value = pow(
self.pheromone_matrix[current_city][city], self.alpha)
heuristic_value = pow(
1/self.cities_distances[current_city][city], self.beta)
prob = pheromone_value * heuristic_value
probabilities.append(prob)
total_prob += prob
normalized_probs = [p/total_prob for p in probabilities]
selected_index = np.random.choice(len(unvisited_cities),
p=normalized_probs)
next_city = unvisited_cities[selected_index]
return next_city
def update_pheromones(self, tours):
delta_tau = [[0]*self.num_cities for i in range(self.num_cities)]
for tour in tours:
length_of_tour = sum([self.cities_distances[tour[i]][tour[(i + 1)%len(tour)]]
for i in range(len(tour))])
if(length_of_tour < self.best_tour_length):
self.best_tour_length = length_of_tour
self.best_tour = tour
for i in range(len(tour)):
start_city = tour[i]
end_city = tour[(i + 1) % len(tour)]
delta_tau[start_city][end_city] += self.q / \
length_of_tour
for row in range(self.num_cities):
for col in range(self.num_cities):
self.pheromone_matrix[row][col] *= (1-self.rho)
self.pheromone_matrix[row][col] += delta_tau[row][col]
def main():
distances = [
[0, 7, 9, 8],
[7, 0, 10, 4],
[9, 10, 0, 15],
[8, 4, 15, 0]]
aco_solver = AntColonyOptimization(distances, num_ants=10)
iterations = 100
for iteration in range(iterations):
all_tours = []
for ant in range(aco_solver.num_ants):
visited_cities = set()
starting_point = randint(0, aco_solver.num_cities - 1)
path = [starting_point]
while True:
last_visited = path[-1]
available_options = list(set(range(
aco_solver.num_cities)).difference(visited_cities))
chosen_option = aco_solver.select_next_city(last_visited,
available_options)
path.append(chosen_option)
visited_cities.add(chosen_option)
if len(path)==aco_solver.num_cities and chosen_option==starting_point:
break
all_tours.append(path[:-1]) # Exclude the repeated first node at the end.
aco_solver.update_pheromones(all_tours)
print(f'Best Tour Found: {aco_solver.best_tour}')
print(f'Total Distance of Best Tour: {aco_solver.best_tour_length}')
if __name__ == '__main__':
main()
```
阅读全文
相关推荐




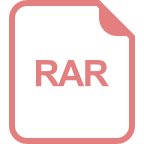











