用python解决: 3.格式转换:请将提供的demo.fastq格式文件中的序列转换为fasta文件格式输出
时间: 2024-09-23 21:16:56 浏览: 105
在Python中,我们可以使用生物信息学库如`biopython`来处理FASTQ和FASTA格式的序列数据。以下是一个简单的示例,说明如何读取fastq文件并将其内容转换为fasta格式,并保存到一个新的文件中:
```python
from Bio import SeqIO
# 假设我们有一个名为demo.fastq的文件
input_file = "demo.fastq"
output_file = "demo.fasta"
# 使用SeqIO模块的parse()函数读取fastq文件
with open(input_file, 'r') as in_fh:
seqs = SeqIO.parse(in_fh, 'fastq')
# 将每个sequence转换为fasta格式并写入新文件
with open(output_file, 'w') as out_fh:
SeqIO.write(seqs, out_fh, 'fasta')
print(f"Fastq data has been converted and saved to {output_file}.")
相关问题
格式转换:请将提供的demo.fastq格式文件中的序列转换为fasta文件格式输出.
将FastQ格式文件转换为FASTA格式文件通常涉及到读取原始文件并提取DNA/RNA序列信息,然后将其格式调整为FASTA的标准格式。这里是一个简单的Python示例,使用Biopython库来完成这个任务:
```python
from Bio import SeqIO
def fastq_to_fasta(input_file, output_file):
with open(input_file, 'r') as fastq_handle, open(output_file, 'w') as fasta_handle:
for record in SeqIO.parse(fastq_handle, 'fastq'):
# 提取序列和质量字符串
seq = str(record.seq)
qual = record.letter_annotations.get('phred_quality', '')
# FASTA格式的每一行只包含序列表达
fasta_record = f">{record.description}\n{seq}\n"
fasta_handle.write(fasta_record)
# 使用示例
input_fastq = "demo.fastq"
output_fasta = "demo.fasta"
fastq_to_fasta(input_fastq, output_fasta)
格式转换:请将提供的demo.fastq格式文件中的序列转换为fasta文件格式输出
格式转换通常涉及到文本数据结构的转换,特别是在生物信息学领域,如从FastQ到FASTA。FastQ是一种常见的基因测序数据存储格式,每个条目包含四行:序号、质量标签、序列本身和附加的质量值。而FASTA则是一个更简洁的格式,仅包含两条信息:一个标识符(序列名称)和该序列。
如果你想要手动完成这个转换,可以编写一些简单的脚本,例如Python,通过读取FastQ文件,逐行提取序列信息并替换每行的格式:
```python
def fastq_to_fasta(input_file, output_file):
with open(input_file, 'r') as in_file, open(output_file, 'w') as out_file:
for line_num, line in enumerate(in_file, start=1):
if line_num % 4 == 1: # 如果是序列行
identifier = f">{line.strip()[1:].split()[0]}\n" # 提取序列ID
sequence = line.strip()[7:] + "\n" # 提取序列内容
elif line_num % 4 == 0: # 如果是加质量值行,跳过
continue
else:
pass # 跳过余下质量值行
out_file.write(identifier)
out_file.write(sequence)
# 使用函数
fastq_to_fasta("demo.fastq", "output.fasta")
```
运行上述代码后,`demo.fastq`文件的内容就会被转换并保存为新的`output.fasta`文件。
阅读全文
相关推荐
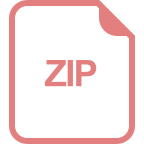
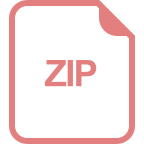
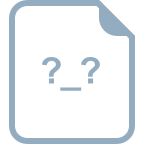
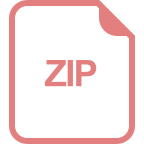
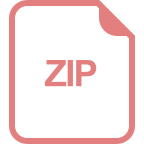
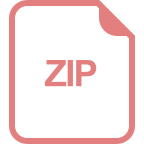
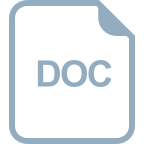

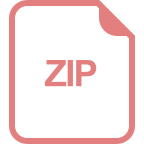
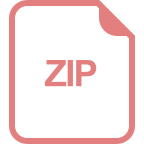
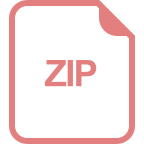
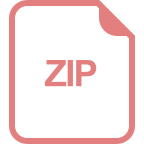
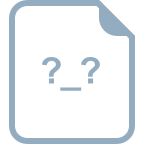



