C语言:输入一个年份,生成该年的月份数组,再输入一个月份号,输出该月的天数(考虑是否为闰年)
时间: 2024-12-14 09:13:42 浏览: 5
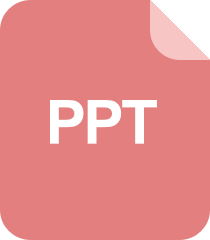
字符数组的输入输出-C语言数组教学
在C语言中,你可以创建一个函数来处理这个需求。首先,你需要判断输入的年份是否为闰年,然后生成对应月份的天数数组。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdbool.h>
// 判断是否为闰年
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 == 0)
return year % 400 == 0;
else
return true;
}
// 生成并返回月份天数数组
int* get_days_in_month(int year, int month) {
static int days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 非闰年的月份天数
if (is_leap_year(year)) {
days[1] = 29; // 闰年时,二月有29天
}
return days + month - 1; // 从数组起始位置偏移得到指定月份的天数指针
}
int main() {
int year, month;
printf("请输入一个年份:");
scanf("%d", &year);
printf("请输入一个月份(1-12):");
scanf("%d", &month);
int* days_for_month = get_days_in_month(year, month);
printf("月份 %d 的天数是:%d\n", month, *days_for_month);
return 0;
}
```
在这个程序中,`get_days_in_month` 函数会根据年份的判断返回相应的月份天数。在`main`函数中,用户会被提示输入年份和月份,然后程序会输出相应月份的天数。
阅读全文
相关推荐
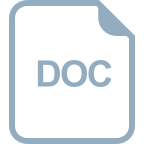
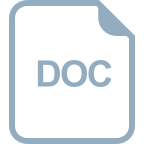
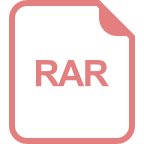
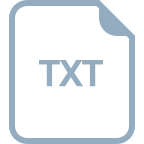
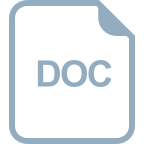
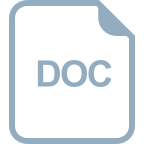
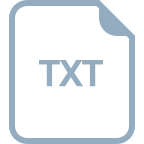
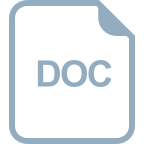
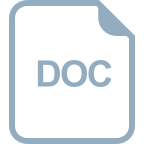
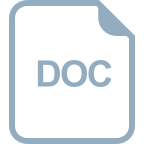
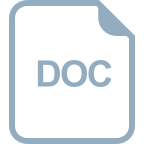
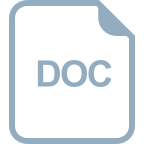
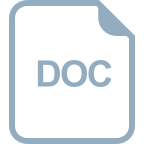
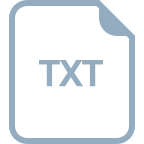
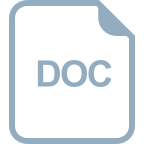
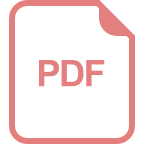
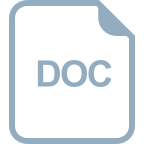
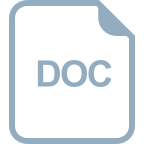