如何在Visual Studio Code (VSCode) 中编写一个简单的程序,用于将用户输入的学号逆序显示?
时间: 2024-10-19 18:12:29 浏览: 8
在 Visual Studio Code (VSCode) 中创建一个简单的命令行应用程序,可以使用 Python 编程语言。下面是一个步骤说明:
1. **打开VSCode**:启动VSCode并创建一个新的文件,例如 "reverse_student_id.py"。
2. **编写代码**:
```python
def reverse_student_id(input_string):
return input_string[::-1]
# 获取用户输入
student_id = input("请输入学生的学号:")
reversed_id = reverse_student_id(student_id)
print(f"学号逆序后为:{reversed_id}")
```
在这个程序中,我们首先定义了一个函数 `reverse_student_id` 来逆序字符串,然后通过 `input()` 函数获取用户的输入作为学号,最后打印出逆序后的结果。
3. **运行程序**:
- 在VSCode中,按下 `F5` 或者点击工具栏的 "Run" 按钮,选择 "Run Current File" 来运行程序。
相关问题
visual studio中整数逆序输出
您可以使用以下代码在Visual Studio中实现整数逆序输出:
```C++
#include <iostream>
#include <algorithm>
#include <string>
int main() {
int num;
std::cout << "请输入一个整数:";
std::cin >> num;
std::string str = std::to_string(num);
std::reverse(str.begin(), str.end());
int reversedNum = std::stoi(str);
std::cout << "逆序输出的整数为:" << reversedNum << std::endl;
return 0;
}
```
写一个C语言程序:任意输入一个三位整数,然后逆序输出。要求输入本人学号后三位完成运行。
下面是一个简单的C语言程序,它会提示用户输入一个三位整数,并将其逆序输出:
```c
#include <stdio.h>
int main() {
int number, reversed_number = 0;
// 提示用户输入学号后三位并转换成整数
printf("请输入你的学号后三位整数: ");
scanf("%d", &number);
// 确保输入的是三位数
if (number < 100 || number > 999) {
printf("输入的数字应为三位。\n");
return 1; // 如果不是三位数,退出程序
}
// 逆序计算
while (number != 0) {
reversed_number = reversed_number * 10 + number % 10;
number /= 10;
}
// 输出逆序后的数字
printf("逆序后的数字是: %d\n", reversed_number);
return 0;
}
```
当你运行这个程序时,按照提示输入你的学号后三位数字,比如123,程序就会输出321。
阅读全文
相关推荐
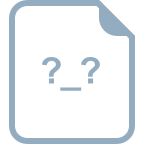
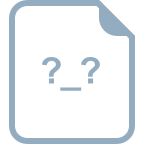
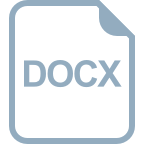
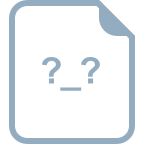
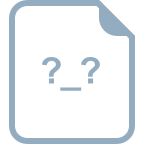
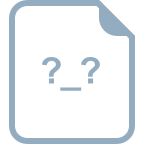
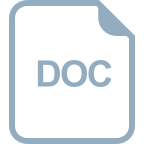
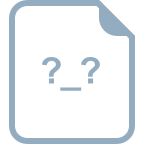
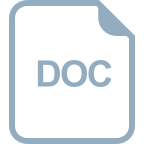
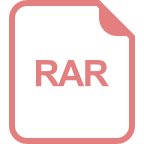