如何在C# WinForms中实现一个功能,即先让绘制的矩形框以30度角旋转,然后再沿该30度角度线进行直线移动?
时间: 2024-10-25 08:12:51 浏览: 23
在C# WinForms中,为了实现一个矩形框以30度角旋转并沿旋转后的30度角度线直线移动的功能,你需要利用Graphics类和Transformations类提供的API。以下是大致步骤:
1. **创建一个矩形控件**:
首先,在WinForms项目中添加一个PictureBox控件,用于显示你的矩形。
2. **设置初始位置**:
设置PictureBox的位置和大小,以便显示一个矩形。
3. **事件处理**:
在PictureBox的MouseMove事件上添加处理程序,这个函数会在鼠标移动时触发。
4. **图形变换**:
使用`Graphics.TranslateTransform`将矩形平移,并结合`Graphics.RotateTransform`进行旋转。例如,你可以这样做:
```csharp
private void pictureBox_MouseMove(object sender, MouseEventArgs e)
{
// 获取当前鼠标位置
Point mousePosition = new Point(e.X, e.Y);
// 创建新的图形上下文
Graphics g = pictureBox.CreateGraphics();
// 清空之前的变换
g.Transform ResetTransform();
// 定义旋转中心点(通常为PictureBox的中心)
Point rotateCenter = pictureBox.ClientRectangle.Center;
// 计算30度旋转后的坐标
PointF rotatedPoint = new PointF(mousePosition.X - rotateCenter.X, mousePosition.Y - rotateCenter.Y);
float rotationAngleInRadians = Math.PI / 180 * 30;
PointF rotatedMousePos = new PointF(rotatedPoint.X * Math.Cos(rotationAngleInRadians) - rotatedPoint.Y * Math.Sin(rotationAngleInRadians),
rotatedPoint.X * Math.Sin(rotationAngleInRadians) + rotatedPoint.Y * Math.Cos(rotationAngleInRadians));
// 旋转矩形
g.RotateTransform(rotationAngleInDegrees, rotateCenter);
// 移动到旋转后的坐标
g.TranslateTransform(rotatedMousePos.X, rotatedMousePos.Y);
// 绘制矩形(这里假设矩形的宽度和高度已知)
Pen pen = new Pen(Color.Black, 2);
RectangleF rect = new RectangleF(rotateCenter.X - rectangleWidth / 2, rotateCenter.Y - rectangleHeight / 2,
rectangleWidth, rectangleHeight);
g.DrawRectangle(pen, rect);
// 释放图形资源
g.Dispose();
}
```
5. **最后记得关闭窗口时清除缓存**:
在FormClosing事件中添加清理代码,避免内存泄漏。
阅读全文
相关推荐
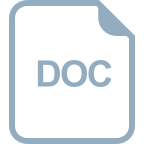
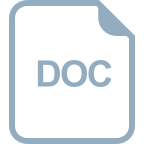
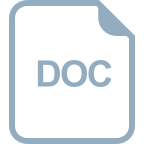
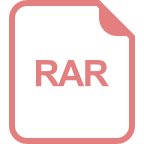
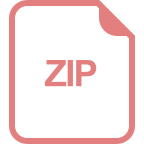
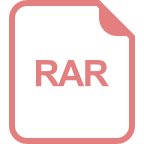
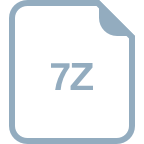
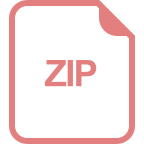
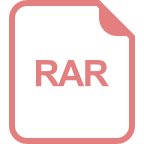
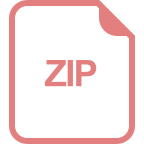
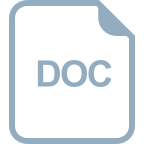
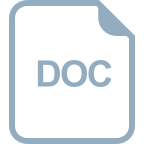
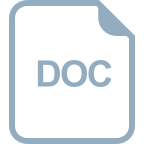
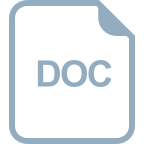
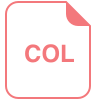
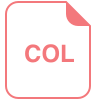
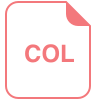
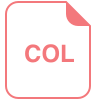

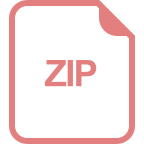