如何使用Python编程语言有效地从网站上下载PDF文件?
时间: 2024-12-01 12:30:36 浏览: 7
在Python中,可以使用一些库来高效地下载网站上的PDF文件,如`requests`库用于获取网页内容,`beautifulsoup4`或`lxml`库用于解析HTML,找到PDF链接,然后使用`wget`、`pdfkit`或者`PyPDF2`等库进行下载。以下是一个简单的步骤示例:
1. **安装所需库**:
首先,确保已经安装了`requests`, `beautifulsoup4` 和`wget`(如果使用wget而非Python内置下载)。如果没有,可以分别通过下面的命令安装:
```
pip install requests beautifulsoup4 wget (or for PyPDF2: pip install pyPDF2)
```
2. **编写脚本**:
使用以下Python代码作为基础模板:
```python
import os
import requests
from bs4 import BeautifulSoup
def download_pdf(url):
# 发送GET请求并获取响应
response = requests.get(url)
# 检查请求状态码是否成功
if response.status_code == 200:
# 解析HTML内容
soup = BeautifulSoup(response.text, 'html.parser')
# 查找PDF链接
pdf_links = soup.find_all('a', {'href': lambda x: x.endswith(".pdf")})
# 下载每个PDF链接
for link in pdf_links:
pdf_url = link['href']
file_name = os.path.basename(pdf_url) # 获取文件名
save_path = f'downloads/{file_name}' # 定义保存路径
# 如果下载目录不存在,则创建
if not os.path.exists('downloads'):
os.makedirs('downloads')
# 实际下载PDF
with requests.get(pdf_url, stream=True) as req_pdf:
req_pdf.raise_for_status()
with open(save_path, 'wb') as out_file:
for chunk in req_pdf.iter_content(chunk_size=8192):
if chunk: # filter out keep-alive new chunks
out_file.write(chunk)
print(f"下载中... {file_name}")
else:
print(f"无法访问URL: {url}")
# 示例URL替换为你需要下载PDF的网址
download_pdf("http://example.com/your-page")
```
记得将`download_pdf("http://example.com/your-page")`中的URL替换为实际目标网站。
阅读全文
相关推荐
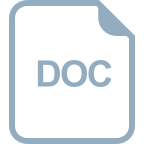
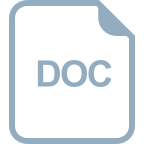
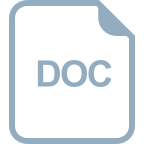
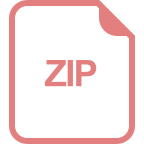
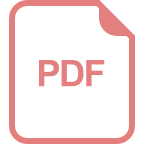
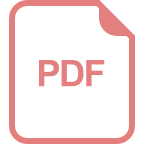
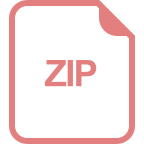
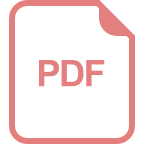
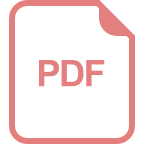
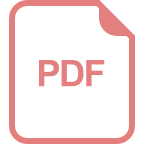
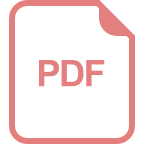
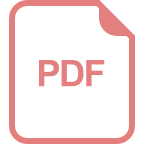
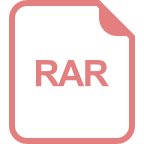
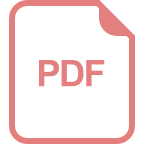
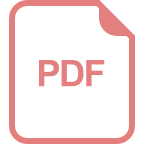
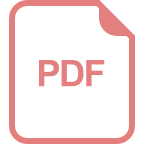
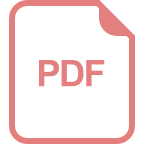
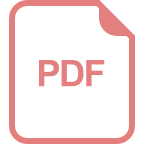
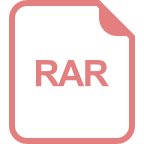