python用excel刷题程序tkinter
时间: 2023-11-01 22:02:49 浏览: 163
Python用Excel刷题程序的实现可以借助tkinter库。首先,我们需要导入`tkinter`和`openpyxl`库,`tkinter`用于创建图形用户界面,`openpyxl`用于读取和操作Excel文件。
1. 创建一个主窗口:
```python
import tkinter as tk
root = tk.Tk()
root.title("Excel刷题程序")
root.geometry("500x500")
```
2. 选择Excel文件:
```python
from tkinter import filedialog
def select_file():
file_path = filedialog.askopenfilename(filetypes=(('Excel files', '*.xlsx'), ('All files', '*.*')))
# 对选择的Excel文件进行处理
# ...
select_file_button = tk.Button(root, text="选择Excel文件", command=select_file)
select_file_button.pack()
```
通过`filedialog.askopenfilename`函数选择Excel文件,并将文件路径存储在`file_path`变量中,接下来可以对该文件进行处理。
3. 读取Excel文件并进行题目展示:
```python
import openpyxl
def read_excel(file_path):
wb = openpyxl.load_workbook(file_path)
ws = wb.active
for row in ws.iter_rows(min_row=2):
question = row[0].value # 假设题目保存在第一列
# 题目处理
print(question)
# 在select_file函数中调用read_excel函数
# ...
read_excel(file_path)
```
使用`openpyxl.load_workbook`函数加载Excel文件,然后通过`wb.active`选择活动的工作表,使用`iter_rows`方法遍历每一行,获取题目的内容并进行处理。
4. 添加答题按钮和答案判定:
```python
def check_answer(index, answer):
# 获取对应行的答案并进行比较
# ...
def show_question(index, question):
question_label = tk.Label(root, text=str(index) + ". " + question)
question_label.pack()
answer_entry = tk.Entry(root)
answer_entry.pack()
check_button = tk.Button(root, text="答题", command=lambda: check_answer(index, answer_entry.get()))
check_button.pack()
```
`check_answer`函数用于获取选择的答案并与正确答案比较。`show_question`函数用于在界面上展示题目和答题选项。
最后,我们需要处理题目的展示顺序和判断答案正确与否的逻辑,这里只展示了基本的框架。根据具体需求,我们还可以添加提交按钮、计分功能等。
以上是一个基于tkinter和openpyxl库实现的Python刷题程序,通过这样的程序,我们可以从Excel文件中读取题目,并在图形界面中展示,并且可以选择答案并进行判定。
阅读全文
相关推荐





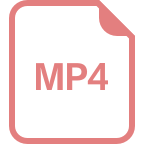









