'application.companyNameCn'通过字符串结构给form.application.companyNameCn赋值
时间: 2024-09-27 20:05:56 浏览: 23
在JavaScript或前端开发中,当你想要通过字符串结构给`form.application.companyNameCn`这个属性赋值时,通常是在处理JSON数据或者是动态设置表单字段。假设你有一个包含公司名称中文的部分的JSON对象,你可以这样做:
```javascript
let jsonData = {
"companyName": {
"en": "Company Name English",
"cn": "公司名称中文"
}
};
// 获取到字符串形式的路径
let path = "application.companyNameCn";
// 使用点符号或数组索引来访问并赋值
let obj = jsonData; // 如果jsonData不在作用域内,需要先解析它
path.split('.').reduce((parent, key) => parent[key], obj) = jsonData["companyName"]["cn"];
```
这行代码会遍历字符串中的`.`分隔符,一步步地深入到`jsonData`对象内部,并最终将`"公司名称中文"`赋值给`form.application.companyNameCn`。
相关问题
post的请求载荷{ids: "2234566"}变成字符串ids: "2234566",requestType: 'form'不可以配置,要怎么请求设置
当你需要将一个JavaScript对象如`{ ids: "2234566" }`转换成URL查询字符串,并且无法直接配置`requestType`为`'form'`,你可以手动构建查询字符串。在Node.js环境中,可以这样做:
```javascript
const obj = { ids: "2234566" };
let queryString = Object.keys(obj).map(key => `${key}=${obj[key]}`).join('&');
// 现在queryString就是 'ids=2234566'
```
如果你想发送这个字符串到服务器作为POST请求(通常用于JSON数据),你需要将其放在`application/x-www-form-urlencoded`编码的数据体中,而不是直接作为URL路径的一部分。这通常涉及到使用`axios`, `fetch`, 或者`jQuery.ajax`这样的库来构造POST请求:
```javascript
const axios = require('axios');
const data = queryString; // 将上面计算出的字符串赋值给data
axios.post('/api/endpoint', data, {
headers: {'Content-Type': 'application/x-www-form-urlencoded'}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
```
import json import requests import sqlite3 import time import threading channel_id = '1114886706903138365' # 连接到SQLite数据库 # 创建本地存储对象 local = threading.local() def get_connection(): # 检查本地存储对象中是否已经创建了连接对象 if not hasattr(local, 'conn'): # 如果没有,则创建连接对象 local.conn =sqlite3.connect('D:/SQLDATA/aaa.db') #连接数据库 return local.conn TOKEN = 'MTExNDA3NDUzMDE1NjEzMDMzNQ.GFKaeX.PVshsn-1qzFCqVBK6RU865CvKld_SUl1f8mP6g' headers={"Content-Type": "application/x-www-form-urlencoded", # 'Authorization':TOKEN, 'authorization': 'OTQwNTc3NzUyODEyMjM2ODAw.GILEVR.F_Ehv2MQ_lVUNiI3Ip7sGu0TgDKNyOyswcPnv8' } req = requests.get( 'https://discordapp.com/api/v9/channels/1070425809321480272/messages?limit=50', headers=headers) resss = req.content.decode('utf-8') data = json.loads(resss) def getcontent(): # 获取连接对象 conn = get_connection() # 创建游标对象 c = conn.cursor() for article in data: # 内容 id=article['id'] content = article['content'] # 频道ID channel_id = article['content'] # author author_id = article['author']['id'] author_name = article['author']['username'] timestamp = article['timestamp'] c.execute( "INSERT OR IGNORE INTO getData (Id,content,channel_id,author_id,author_name,timestamp,nopush) VALUES(:Id,:content,:channel_id,:author_id,:author_name,:timestamp,:nopush)", { "Id": id, "content": content, "channel_id": channel_id, "author_id": author_id, "author_name": author_name, "timestamp": timestamp, "nopush":'1' }, ) # 提交当前事务,保存数据 conn.commit() c.close() # conn.close() print(content) def run_thread(): while True: # 暂停 0.5 秒钟 time.sleep(0.5) # 创建一个线程,执行 do_something 函数 threading.Thread(target=getcontent).start() if __name__ == '__main__': run_thread() 错误在哪
这段代码存在以下几个错误:
1. 在导入模块时,应该使用换行符将不同的导入语句分开。
2. 在函数 `getcontent()` 中的 `for` 循环缺少缩进。
3. 在函数 `getcontent()` 中,变量 `channel_id` 的赋值应该改为 `channel_id = article['channel_id']`。
4. 在函数 `getcontent()` 中,变量 `nopush` 的赋值应该改为 `nopush': 1`,即不需要使用字符串。
5. 在函数 `getcontent()` 中,游标对象 `c` 在循环中被关闭,应该将其移至循环外。
6. 在函数 `run_thread()` 中,创建新线程时应该将函数名 `getcontent` 改为 `get_content`。
7. 在函数 `run_thread()` 中,应该将连接对象 `conn` 的关闭语句放在 `while` 循环的外面。
修改后的代码如下:
```python
import json
import requests
import sqlite3
import time
import threading
channel_id = '1114886706903138365'
# 连接到SQLite数据库
# 创建本地存储对象
local = threading.local()
def get_connection():
# 检查本地存储对象中是否已经创建了连接对象
if not hasattr(local, 'conn'):
# 如果没有,则创建连接对象
local.conn = sqlite3.connect('D:/SQLDATA/aaa.db')
# 连接数据库
return local.conn
TOKEN = 'MTExNDA3NDUzMDE1NjEzMDMzNQ.GFKaeX.PVshsn-1qzFCqVBK6RU865CvKld_SUl1f8mP6g'
headers = {
"Content-Type": "application/x-www-form-urlencoded",
"authorization": "OTQwNTc3NzUyODEyMjM2ODAw.GILEVR.F_Ehv2MQ_lVUNiI3Ip7sGu0TgDKNyOyswcPnv8"
}
req = requests.get('https://discordapp.com/api/v9/channels/1070425809321480272/messages?limit=50', headers=headers)
resss = req.content.decode('utf-8')
data = json.loads(resss)
def get_content():
# 获取连接对象
conn = get_connection()
# 创建游标对象
c = conn.cursor()
for article in data:
# 内容
id = article['id']
content = article['content']
# 频道ID
channel_id = article['channel_id']
# author
author_id = article['author']['id']
author_name = article['author']['username']
timestamp = article['timestamp']
c.execute(
"INSERT OR IGNORE INTO getData (Id,content,channel_id,author_id,author_name,timestamp,nopush) \
VALUES(:Id,:content,:channel_id,:author_id,:author_name,:timestamp,:nopush)",
{
"Id": id,
"content": content,
"channel_id": channel_id,
"author_id": author_id,
"author_name": author_name,
"timestamp": timestamp,
"nopush": 1
},
)
# 提交当前事务,保存数据
conn.commit()
print(content)
c.close()
def run_thread():
while True:
# 暂停 0.5 秒钟
time.sleep(0.5)
# 创建一个线程,执行 do_something 函数
threading.Thread(target=get_content).start()
conn.close()
if __name__ == '__main__':
run_thread()
```
阅读全文
相关推荐
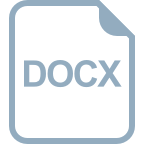
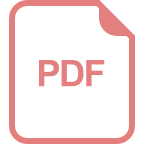
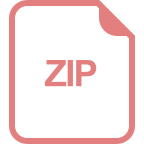
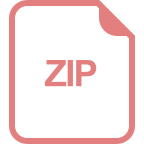
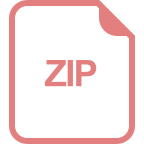
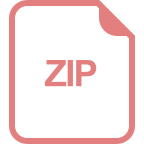
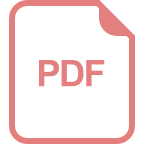
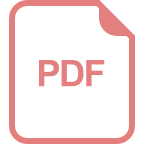
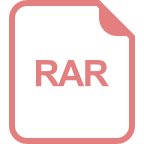
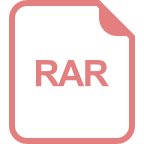
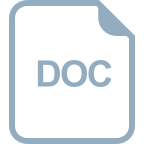
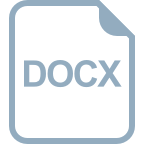
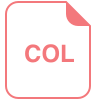
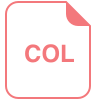
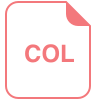
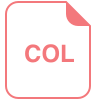
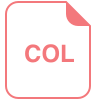