string filepath
时间: 2024-05-26 10:08:07 浏览: 73
string filepath是一个字符串类型的变量,通常用于存储文件路径。在计算机中,每个文件都有一个独特的路径,可以通过该路径来访问该文件。在C++中,string类型可以用来存储文件路径字符串,可以使用标准库中的文件输入输出流和其他相关函数来操作这些文件。
例如,以下是使用string filepath来打开一个文本文件并读取其内容的示例代码:
```
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
string filepath = "example.txt";
ifstream file(filepath);
if (file.is_open())
{
string line;
while (getline(file, line))
{
cout << line << endl;
}
file.close();
}
else
{
cout << "Unable to open file" << endl;
}
return 0;
}
```
相关问题
foreach (string filePath in csvFiles)
`foreach (string filePath in csvFiles)` 是一种 C# 或者其他支持 foreach 循环的语言(如 Python 的 for-in 循环)中的语法结构,用于遍历数组、集合或迭代器返回的序列。在这个上下文中,`csvFiles` 可能是一个字符串类型的数组或列表,包含了多个 CSV 文件的路径。
当这个循环运行时,每次迭代都会将 `filePath` 赋值为 `csvFiles` 中的一个元素,然后执行循环体内的代码。通常,这样的循环会被用于读取每个文件的内容,或者执行针对每个CSV文件的操作,例如解析文件、处理数据等。
举个例子:
```csharp
List<string> csvFiles = new List<string>{"file1.csv", "file2.csv", "file3.csv"};
foreach (string filePath in csvFiles)
{
using (var reader = new StreamReader(filePath))
{
while (!reader.EndOfStream)
{
// 从当前文件行读取数据
var line = reader.ReadLine();
// 处理每一行数据...
}
}
}
```
读取const string filepath中的内容
在C++中,如果你想要读取一个存储在const std::string filepath变量中的文件路径所指向的文件内容,你可以使用标准库中的fstream类。以下是一个简单的例子:
```cpp
#include <fstream>
#include <iostream>
#include <string>
int main() {
const std::string filepath = "example.txt"; // 假设这是你的文件路径
std::ifstream file; // 创建一个ifstream对象
file.open(filepath.c_str()); // 打开文件
if (file.is_open()) { // 检查文件是否成功打开
std::string content((std::istreambuf_iterator<char>(file)), // 读取文件内容到字符串
std::istreambuf_iterator<char>());
file.close(); // 关闭文件
std::cout << content << std::endl; // 输出文件内容
} else {
std::cerr << "无法打开文件:" << filepath << std::endl; // 如果文件无法打开,输出错误信息
}
return 0;
}
```
在这个例子中,我们首先包含了fstream和iostream头文件,分别用于文件操作和输入输出操作。定义了一个const std::string类型变量filepath,它存储了我们想要读取的文件路径。然后,我们创建了一个ifstream对象file,并通过调用其open方法尝试打开文件。如果文件成功打开,我们使用输入迭代器来读取文件的所有内容到一个std::string类型的变量content中,然后输出这个字符串,并关闭文件。如果文件未能打开,我们会输出一个错误信息。
阅读全文
相关推荐
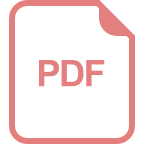
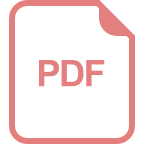
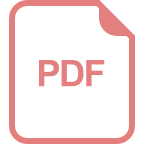













